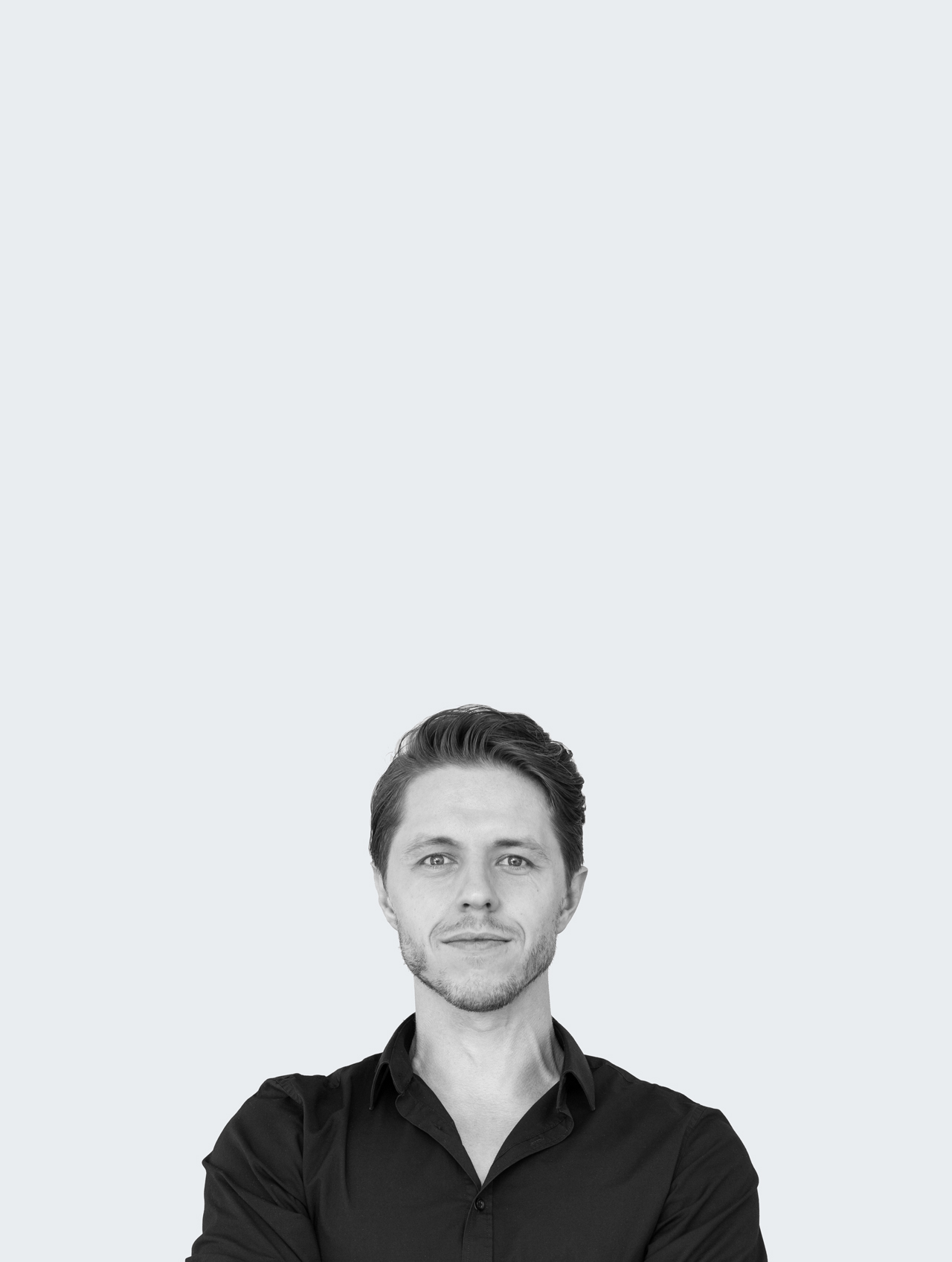
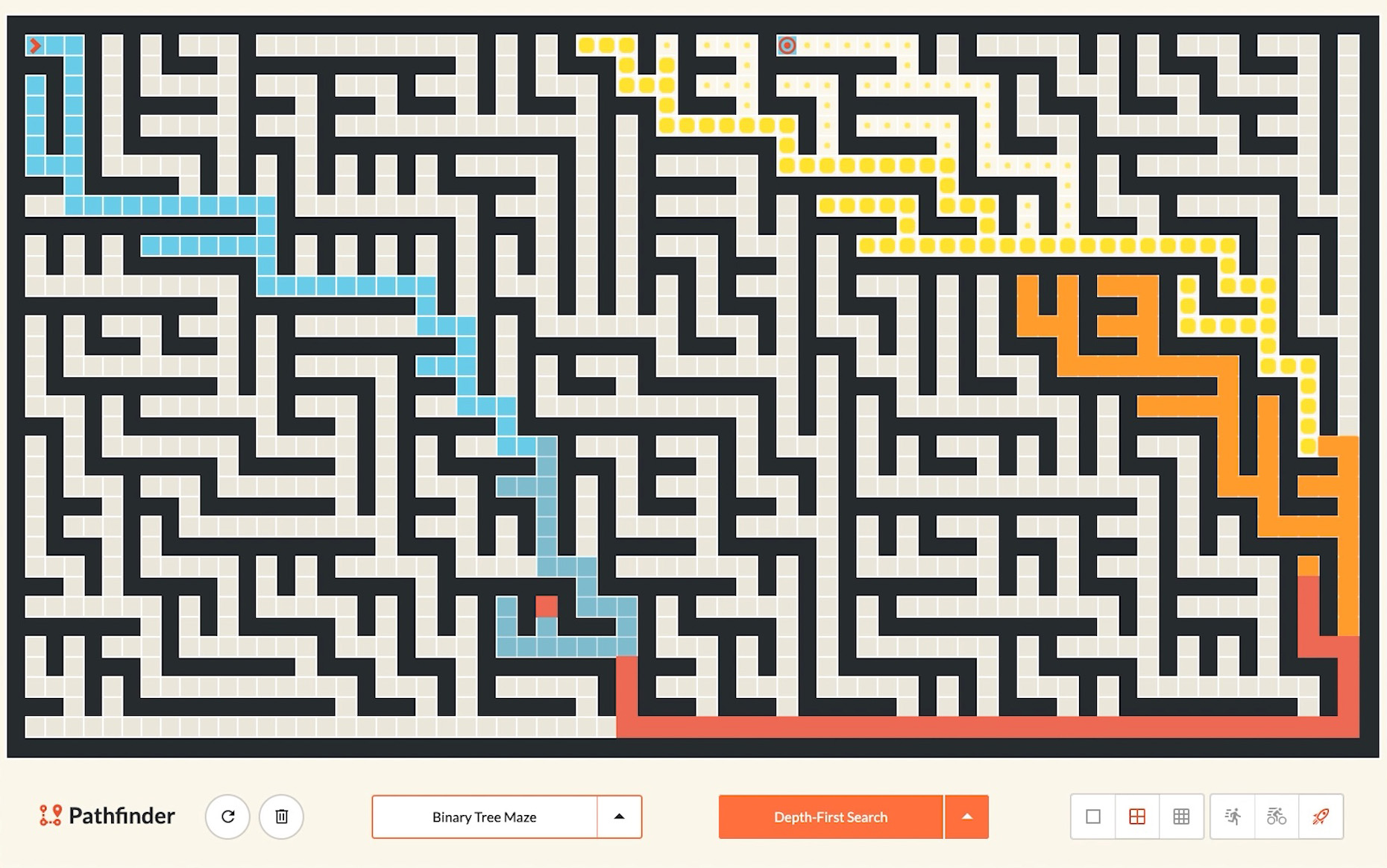
Pathfinder
Vizualize pathfinding algorithms. Build a maze.
Experience six maze generation and five search algorithms.
React · JavaScript · Node.js · CSS · HTML · Material Design · UI · UX
Website ↗Pathfinding Visualizer
Built with Javascript and React Pathfinder visualizes various pathfinding algorithms in action, and more! At its core, a pathfinding algorithm seeks to find the shortest path between two points. After generating or building their own maze users can execute any of the given search algorithms to find the shortest path. The application is interactive, allowing users to reposition start and finish nodes, and this way giving it a playfulness that reassembles a small computer game.UI
User Interface is kept clean and minimal, allowing users to explore all the powerful functionality of the application themselves. In the navigation bar there are two main buttons. One to generate a maze and the other to perform a path search. Icons indicate a few additional but powerful settings including ‘Refresh’ to clean the search paths, ‘Trash-box’ to erase everything including walls, and a few toggle button-groups to resize the grid and change the speed of animation. The main screen space takes a grid, where already are two pointers - the start and finish nodes - ready to be positioned.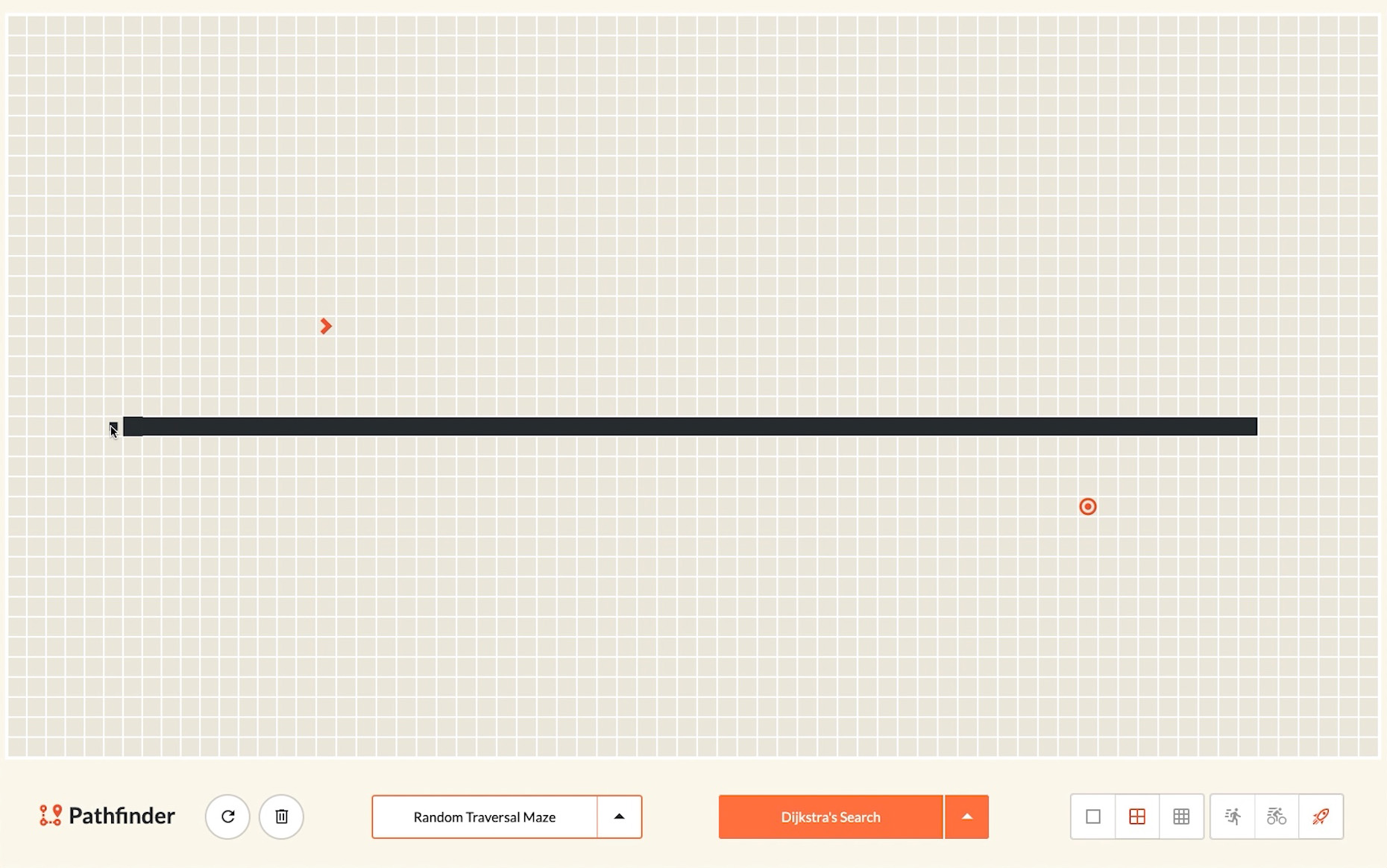
Maze Algorithms
Users are able to draw their own maze over the grid or to generate it using one of the six maze generation algorithms including Random Traversal, Depth-First, Prim’s, Recursive, Binary Tree or Wilson’s Maze.Random Traversal
Random Traversal algorithm, sometimes misleadingly called randomized Prim’s algorithm, is more accurately described as a random traversal. Starting in the upper-left corner, the algorithm keeps an array of the possible directions the maze could be extended (shown in vivid colors). At each step, the maze is extended in one of these random directions, as long as doing so does not reconnect with another part of the maze.
While this algorithm also generates a spanning tree, its behavior is radically different from running Prim’s algorithm on a randomly-weighted graph! Random traversal generates mazes with a very predictable global structure which can be seen in the above the animation.
Random traversal behaves similarly to randomized breadth-first traversal, since typically the maze can only be extended without self-intersecting on a roughly-circular perimeter.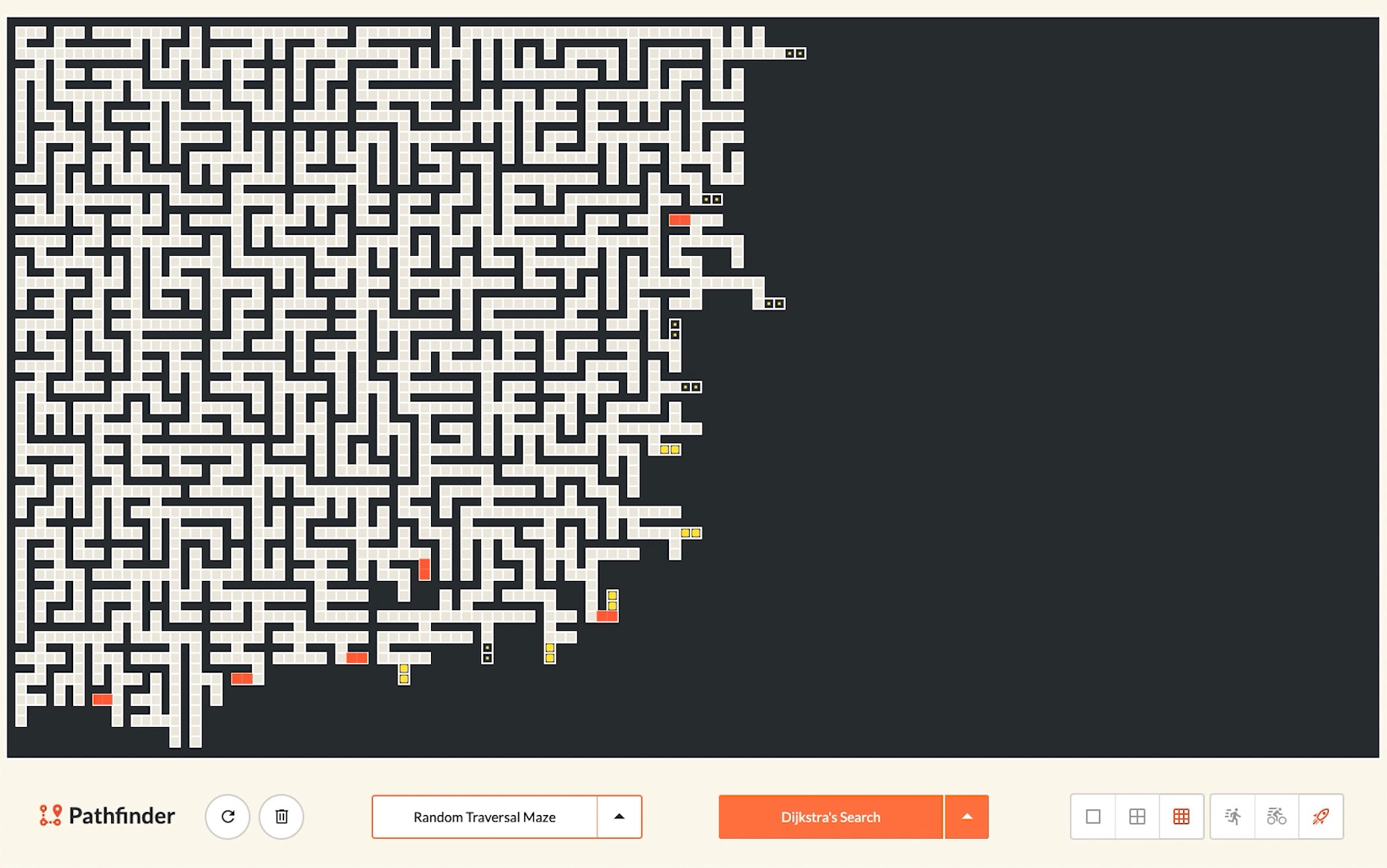
Depth-First Maze
Depth-First Maze generation algorithm uses randomized depth-first traversal. Starting in the upper-left corner, the algorithm keeps an array of the possible directions the maze could be extended (shown in vivid colors). At each step, the maze is extended in a random direction from the previous cell, as long as doing so does not reconnect with another part of the maze.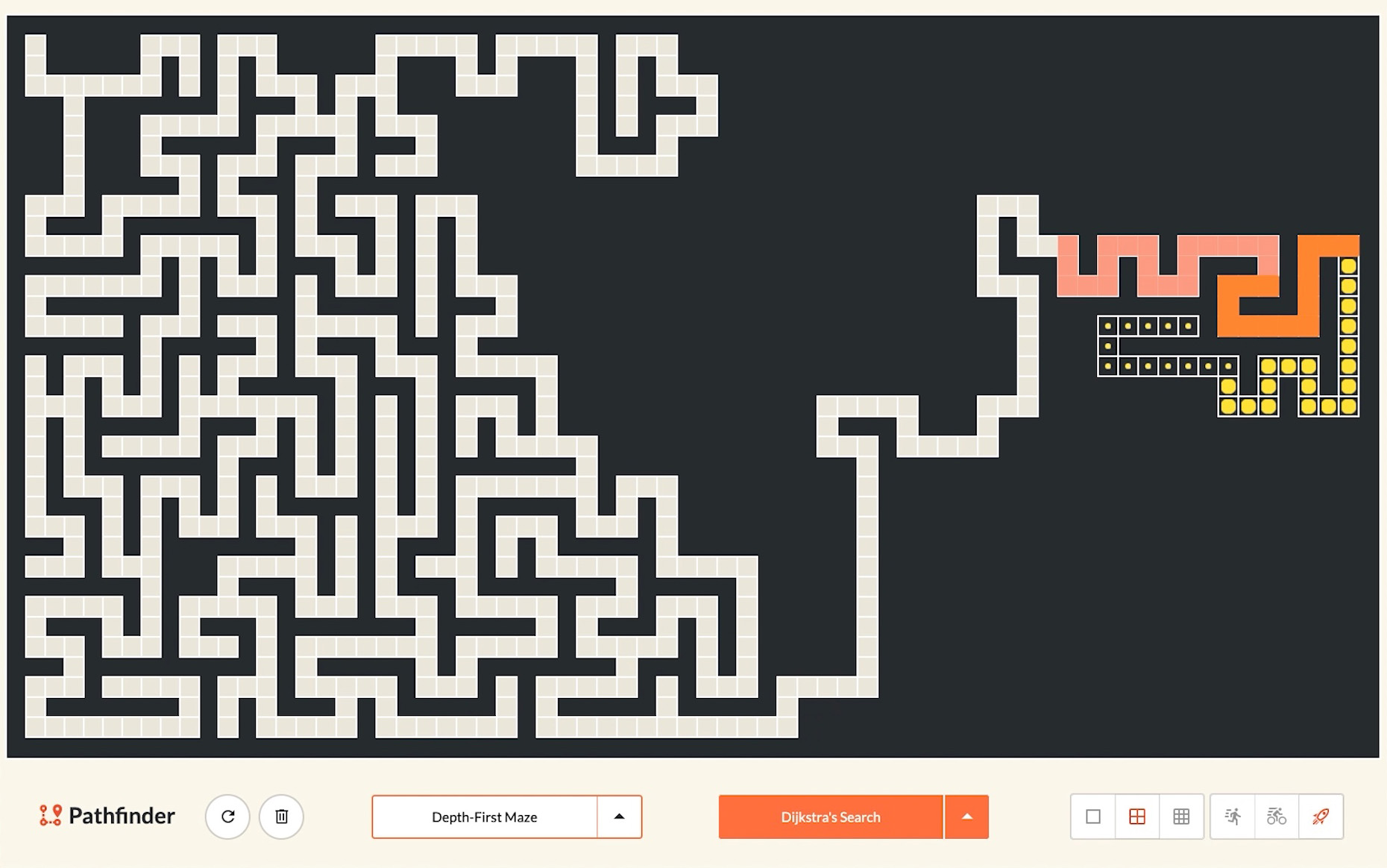
Prim’s Maze
Prim’s algorithm generates a minimum spanning tree from a graph with weighted edges. Starting in the upper-left corner, the algorithm keeps a heap of the possible directions the maze could be extended (shown in vivid colors). At each step, the maze is extended in the direction with the lowest weight, as long as doing so does not reconnect with another part of the maze. Here the edges are initialized with random weights.
Unlike Wilson’s algorithm, this does not result in a uniform spanning tree. Sometimes, random traversal is misleadingly referred to as randomized Prim’s algorithm; however, the two algorithms exhibit radically different behavior!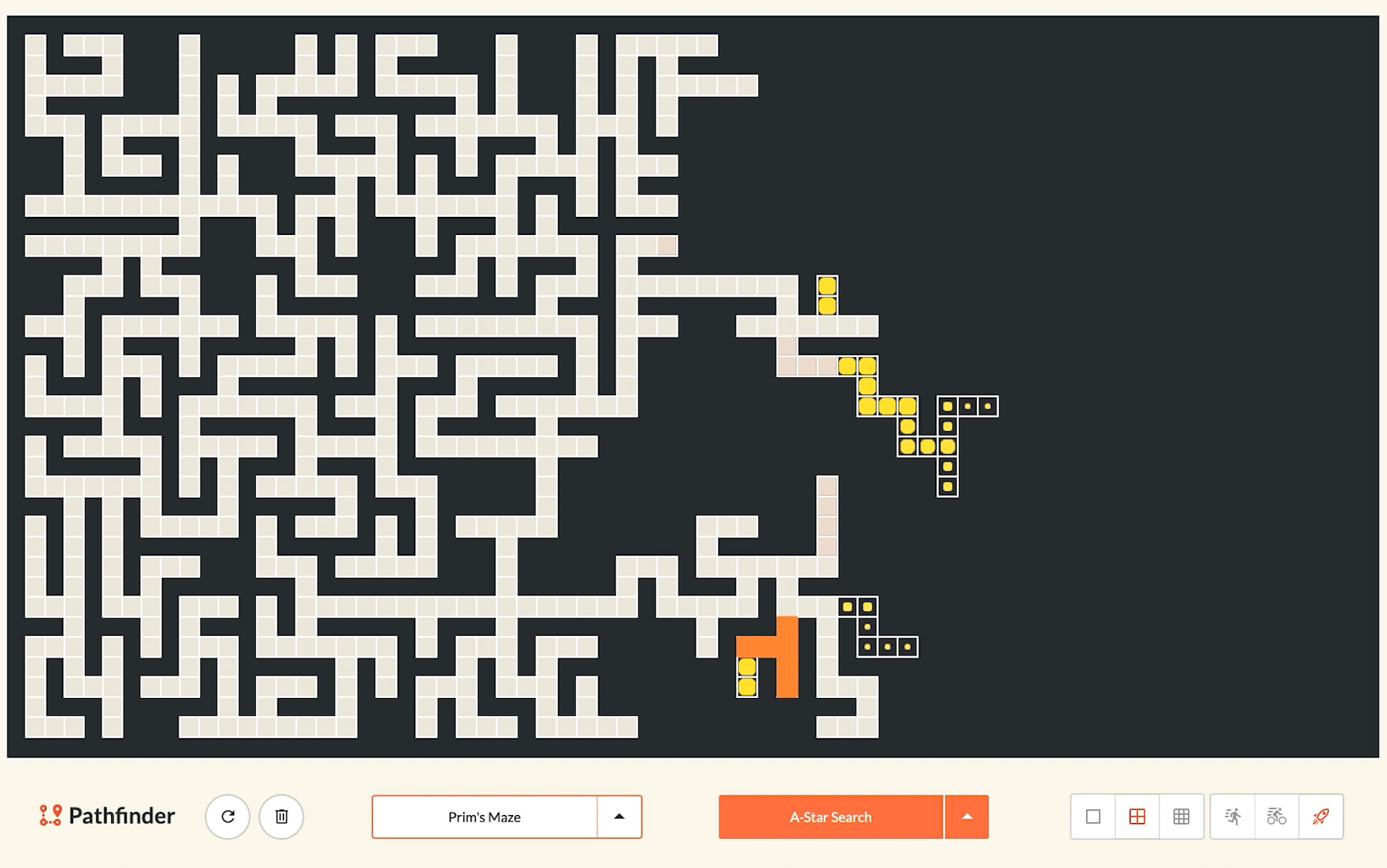
Recursive Maze
Recursive Division Maze Generator is the fastest algorithm without directional biases. This algorithm is particularly fascinating because of its fractal nature: you could theoretically continue the process indefinitely at finer and finer levels of detail.
This algorithm is somewhat similar to recursive backtracking, since they are both stack based, except this focuses on walls instead of passages. As a Wall Builders generator, the process begins with ample space and adds walls until the maze results.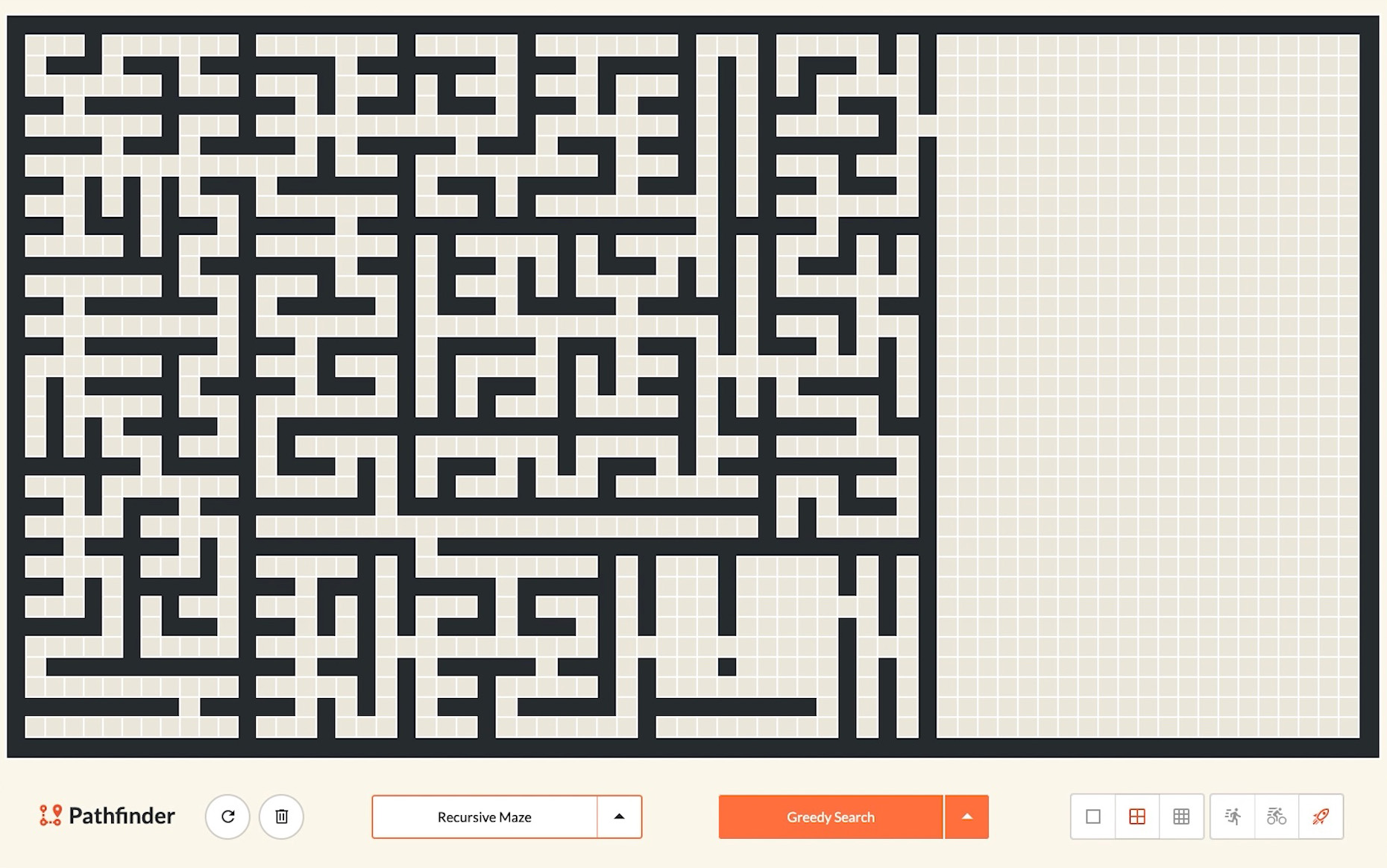
Binary Tree Maze
Binary Tree Maze Generator is one of the very rare algorithms with the ability to generate a perfect maze without keeping any state at all: it is an exact memory-less Maze generation algorithm with no limit to the size of Maze you can create. It can build the entire maze by looking at each cell independently. This is the most straightforward and fastest algorithm possible. Mazes generated are real Binary Tree Data Structure while having a very biased texture.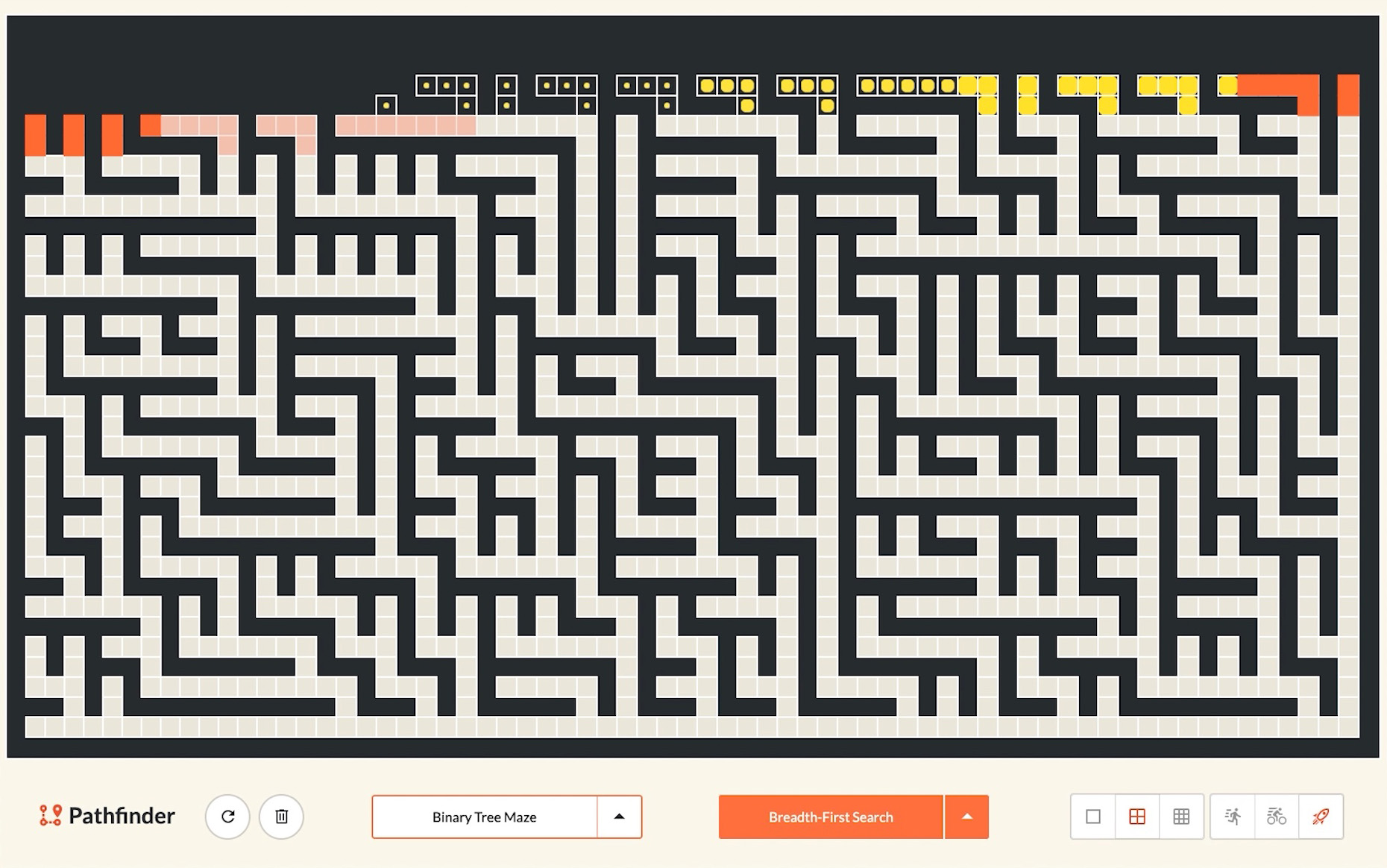
Wilson’s Maze
Wilson’s algorithm uses loop-erased random walks to generate a uniform spanning tree — an unbiased sample of all possible spanning trees. Most other maze generation algorithms, such as Prim’s, random traversal and randomized depth-first traversal, do not have this beautiful property.
The algorithm initializes the maze with an arbitrary starting cell. Then, a new cell is added to the maze, initiating a random walk (shown in vivid colors). The random walk continues until it reconnects with the existing maze. However, if the random walk intersects itself, the resulting loop is erased before the random walk continues.
Initially, the algorithm can be frustratingly slow to watch, as the early random walks are unlikely to reconnect with the small existing maze. However, as the maze grows, the random walks become more likely to collide with the maze and the algorithm accelerates dramatically.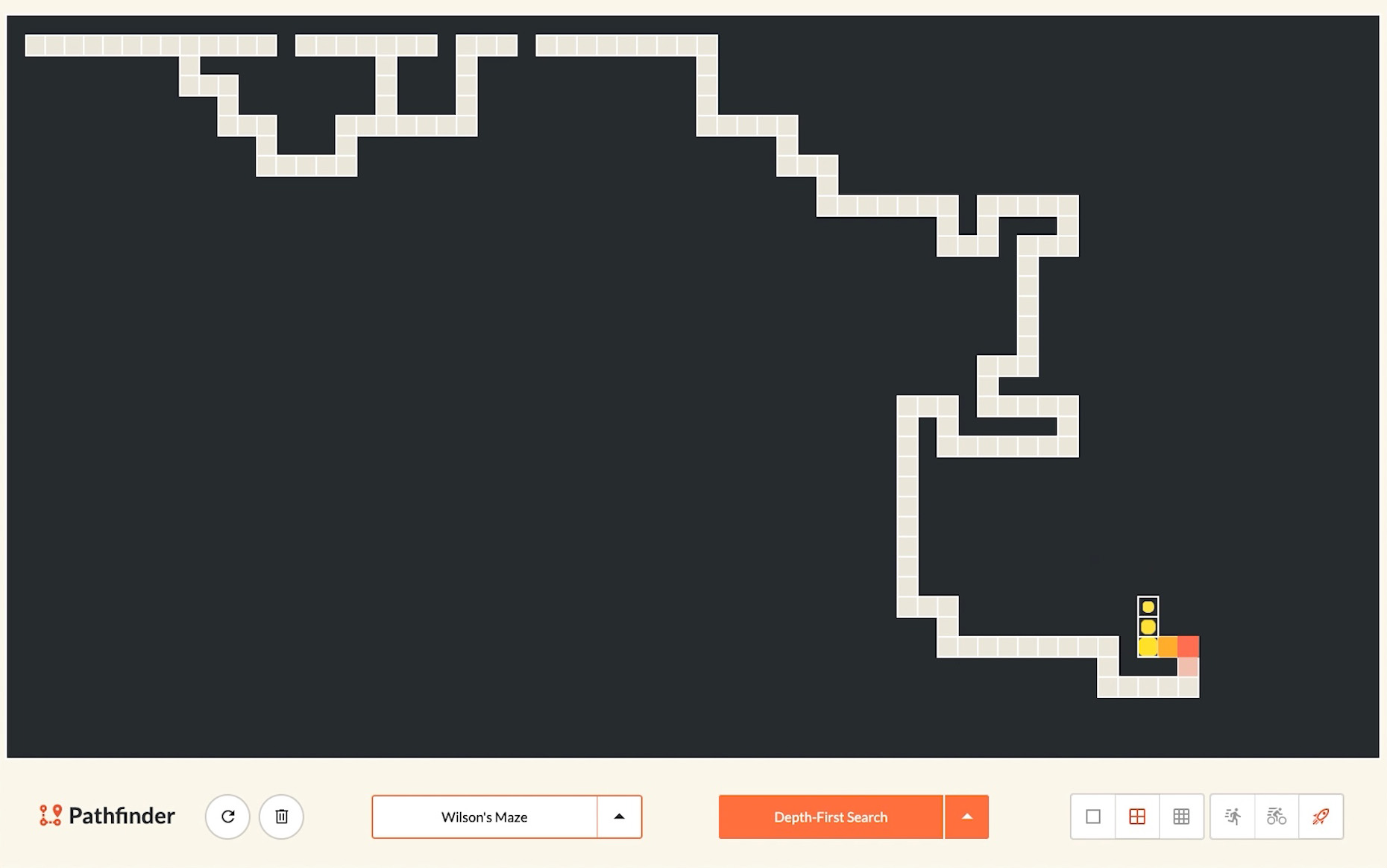
Search Algorithms
Once a maze is created and start as finish nodes are positioned it is time to execute one of the five path search algorithms including Dijkstra’s, A-Star, Greedy, Breadth-First and Depth-First Search.Dijkstra’s Search
Dijkstra's Search is the father of pathfinding algorithms. The algorithm creates a tree of shortest paths from the starting vertex, the source, to all other points in the graph. It is a weighted algorithm and guarantees the shortest path.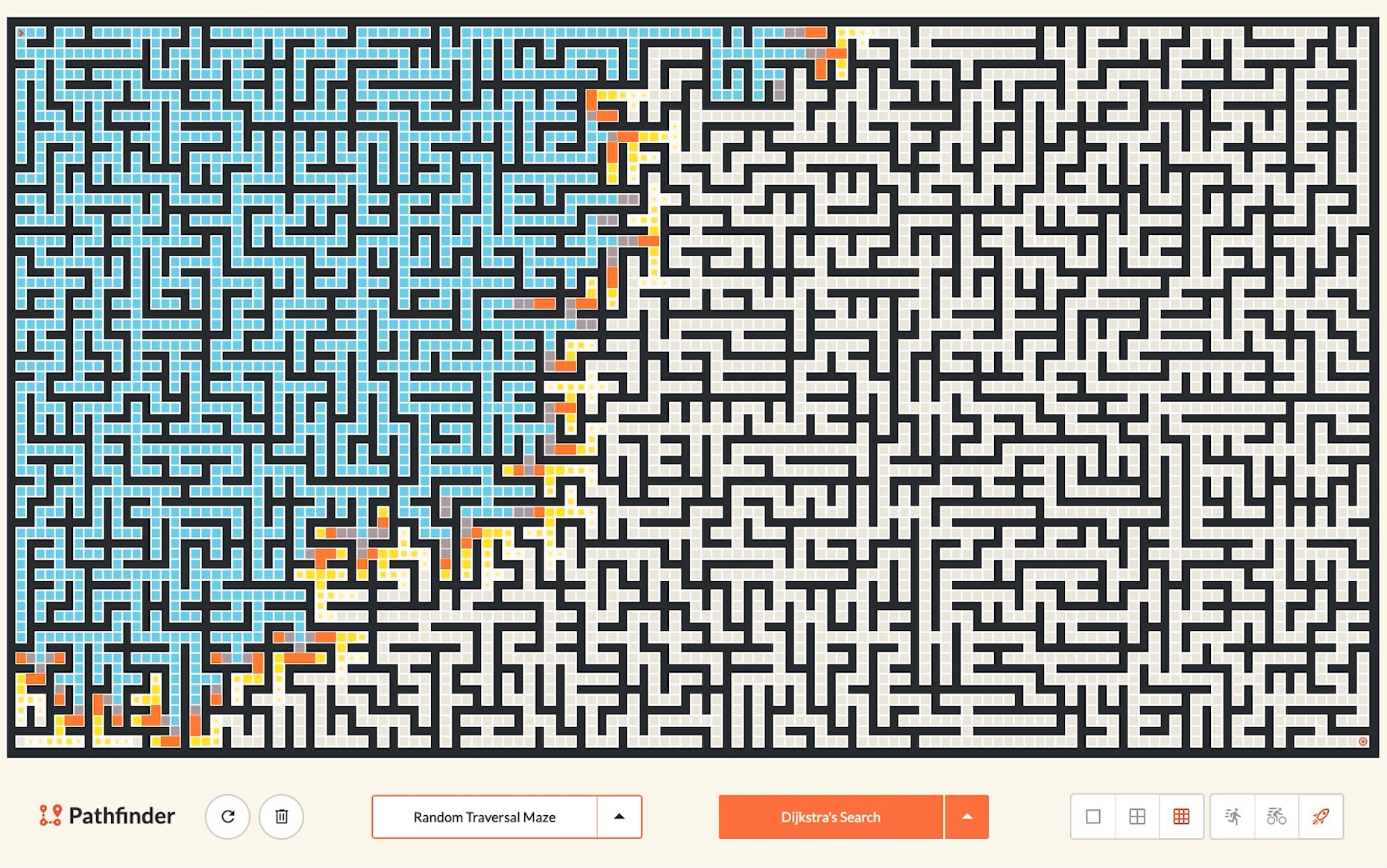
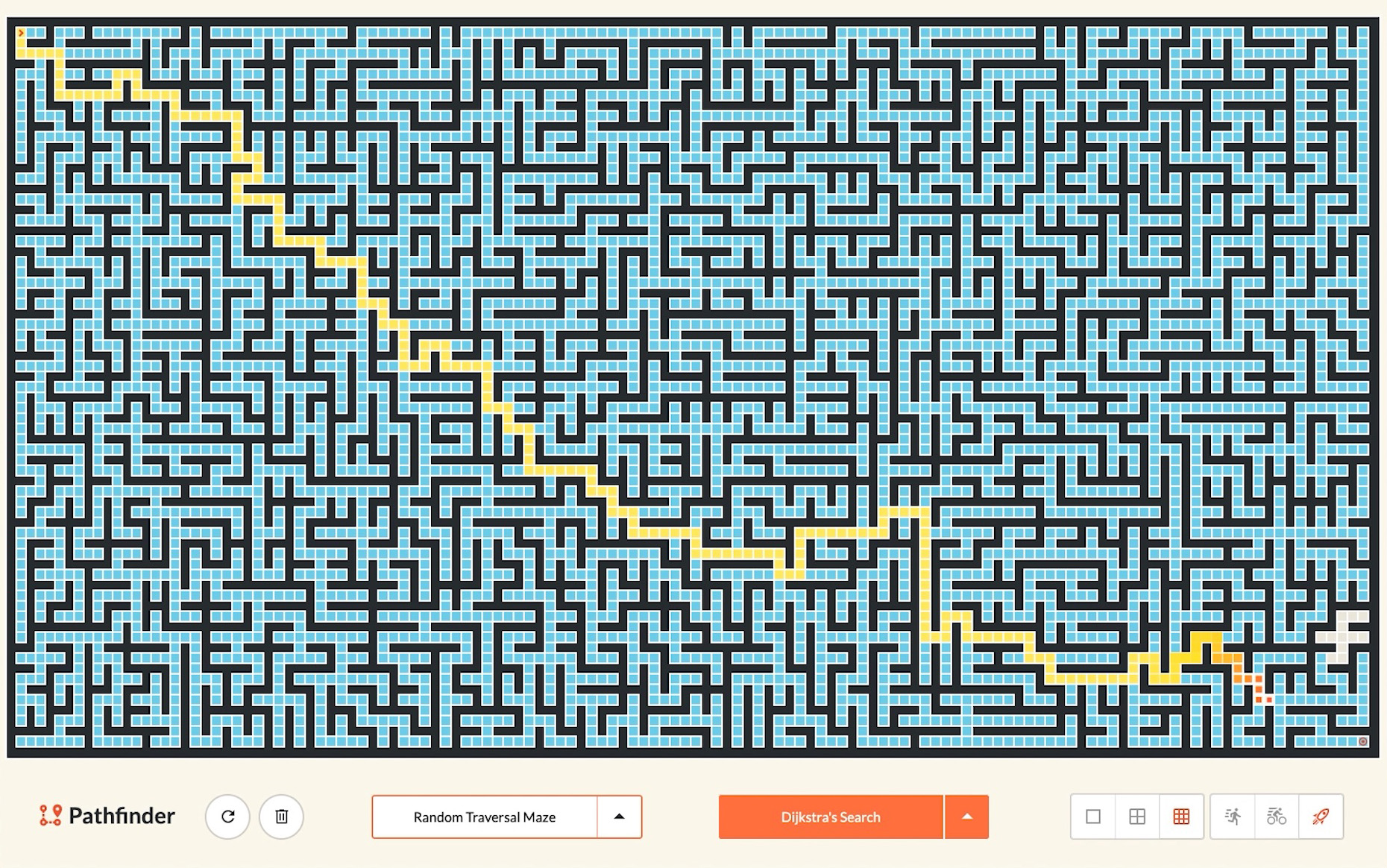
A-Star Search
A* Search is arguably the best pathfinding algorithm. It is weighted and uses heuristics to guarantee the shortest path much faster than Dijkstra's Algorithm. One major practical drawback is its space complexity, as it stores all generated nodes in memory.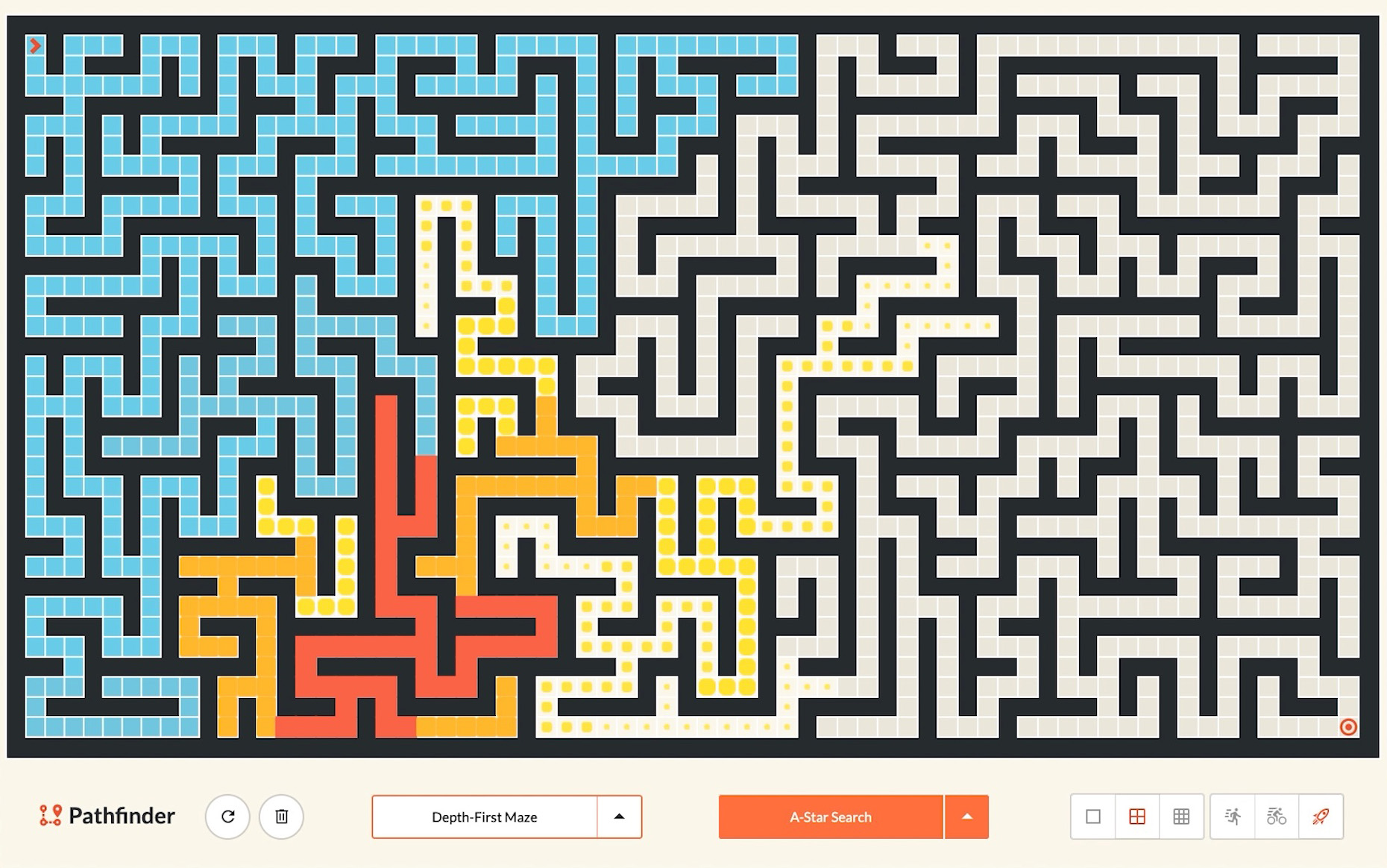
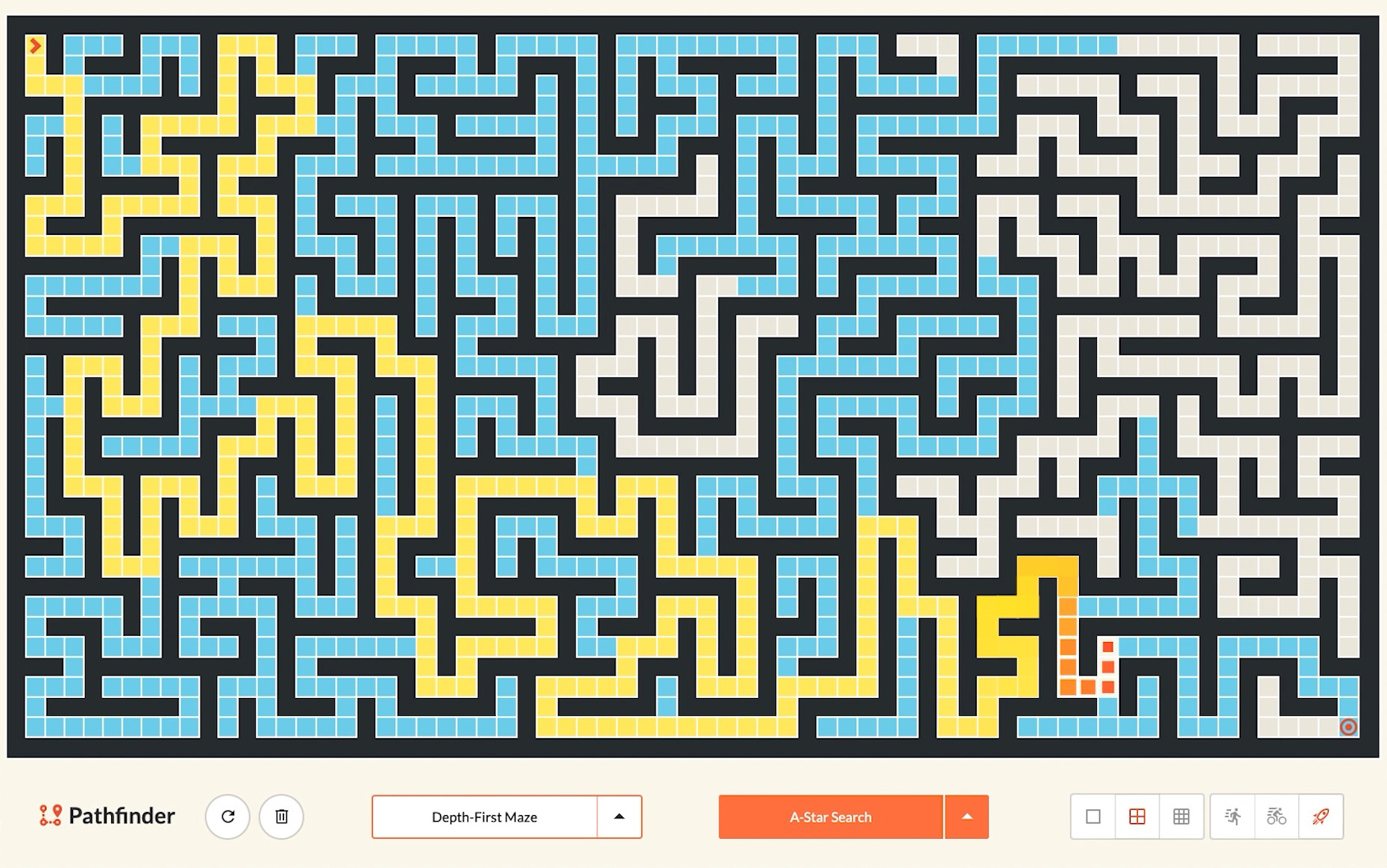
Greedy Search
Greedy Best-first Search is a faster, more heuristic-heavy version of A*. It is weighted and does not guarantee the shortest path.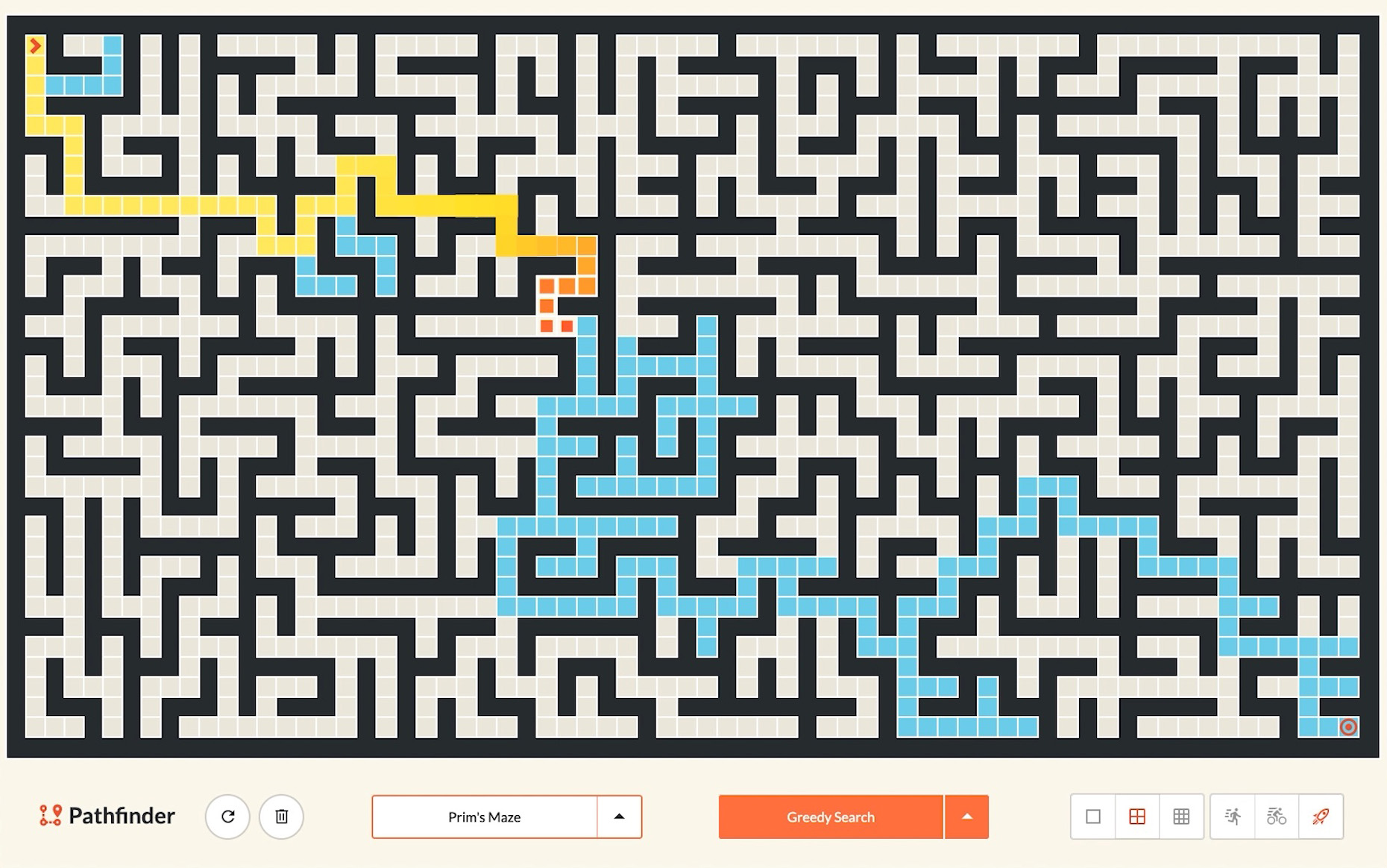
Breadth-First Search
Breath-First Search starts at the tree root, and explores all of the neighbor nodes at the present depth prior to moving on to the nodes at the next depth level. It is unweighted algorithm that guarantees the shortest path.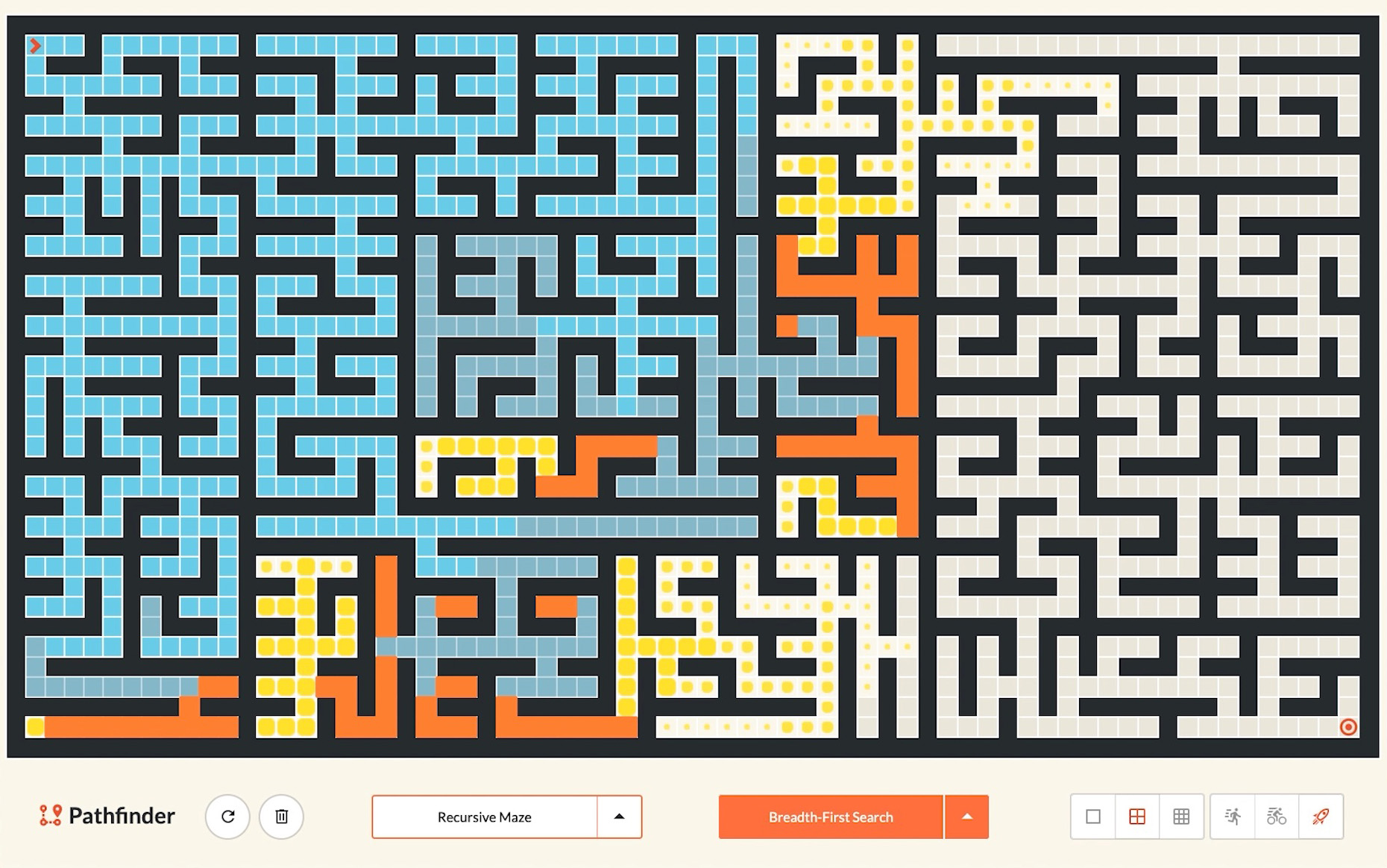
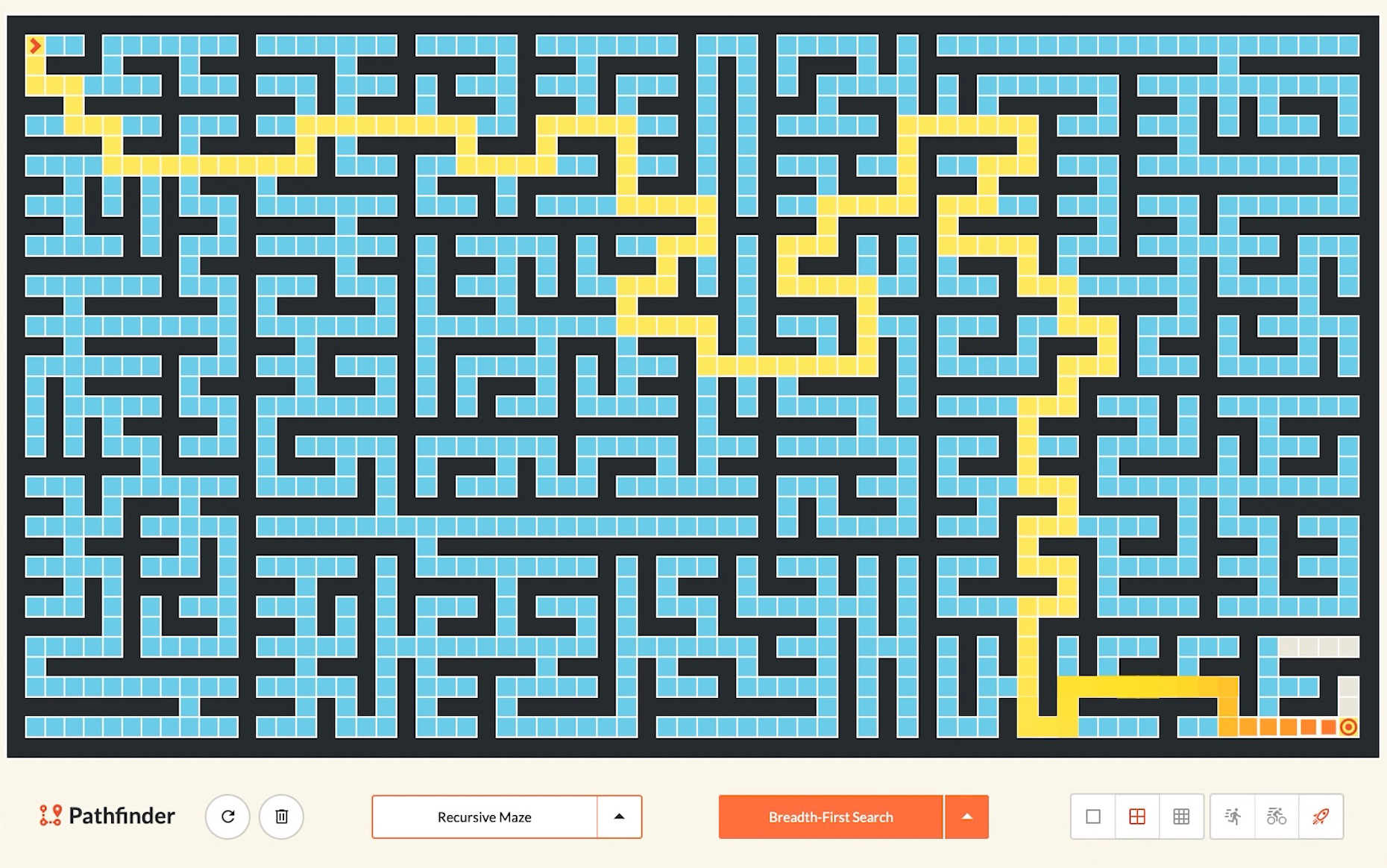
Depth-First Search
Depth-First Search starts at the root node and explores as far as possible along each branch before backtracking. It is unweighted algorithm that does not guarantee the shortest path.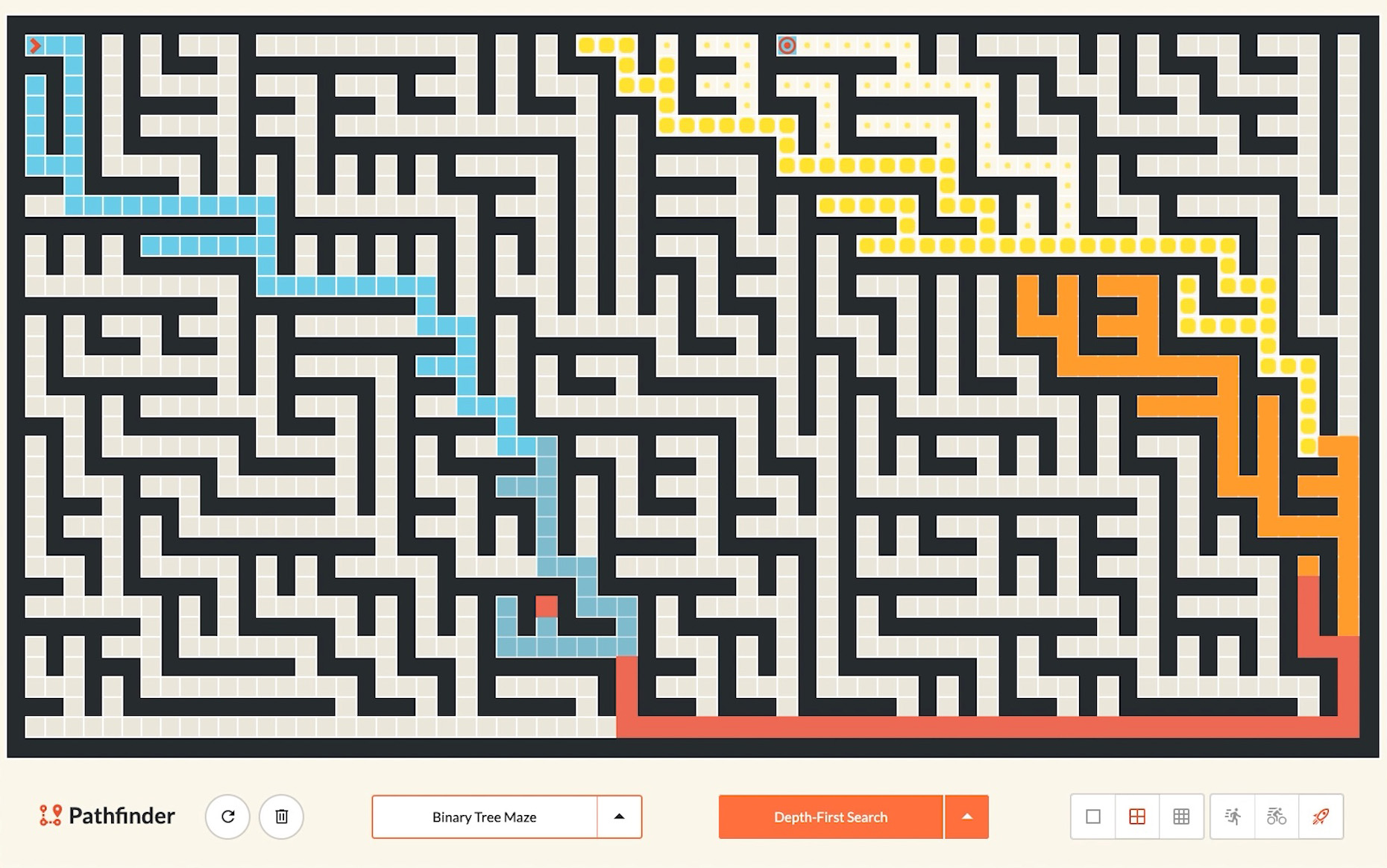
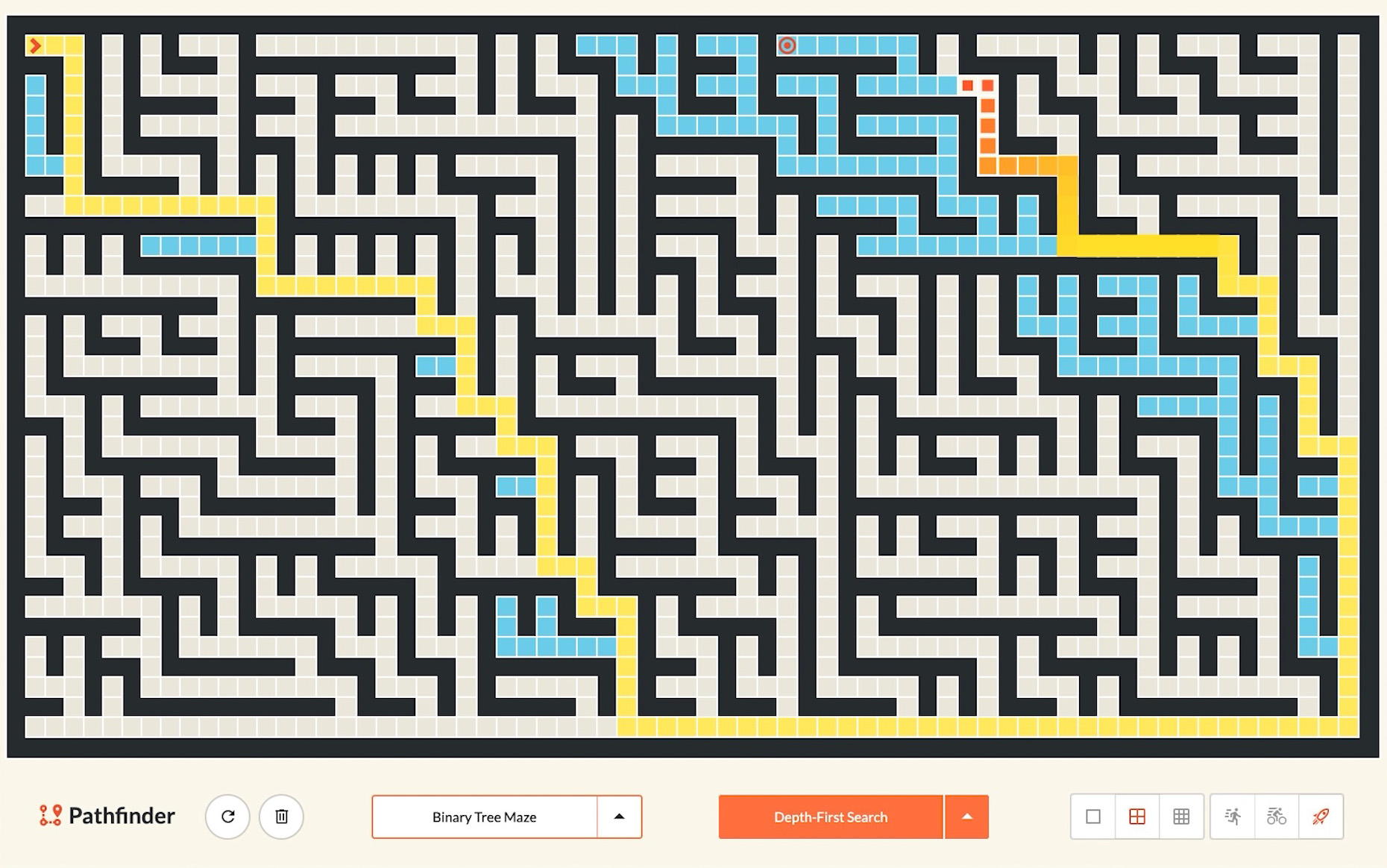
Responsive UI Design
The application is responsive in all screen sizes, including desktops, tablets and smartphones.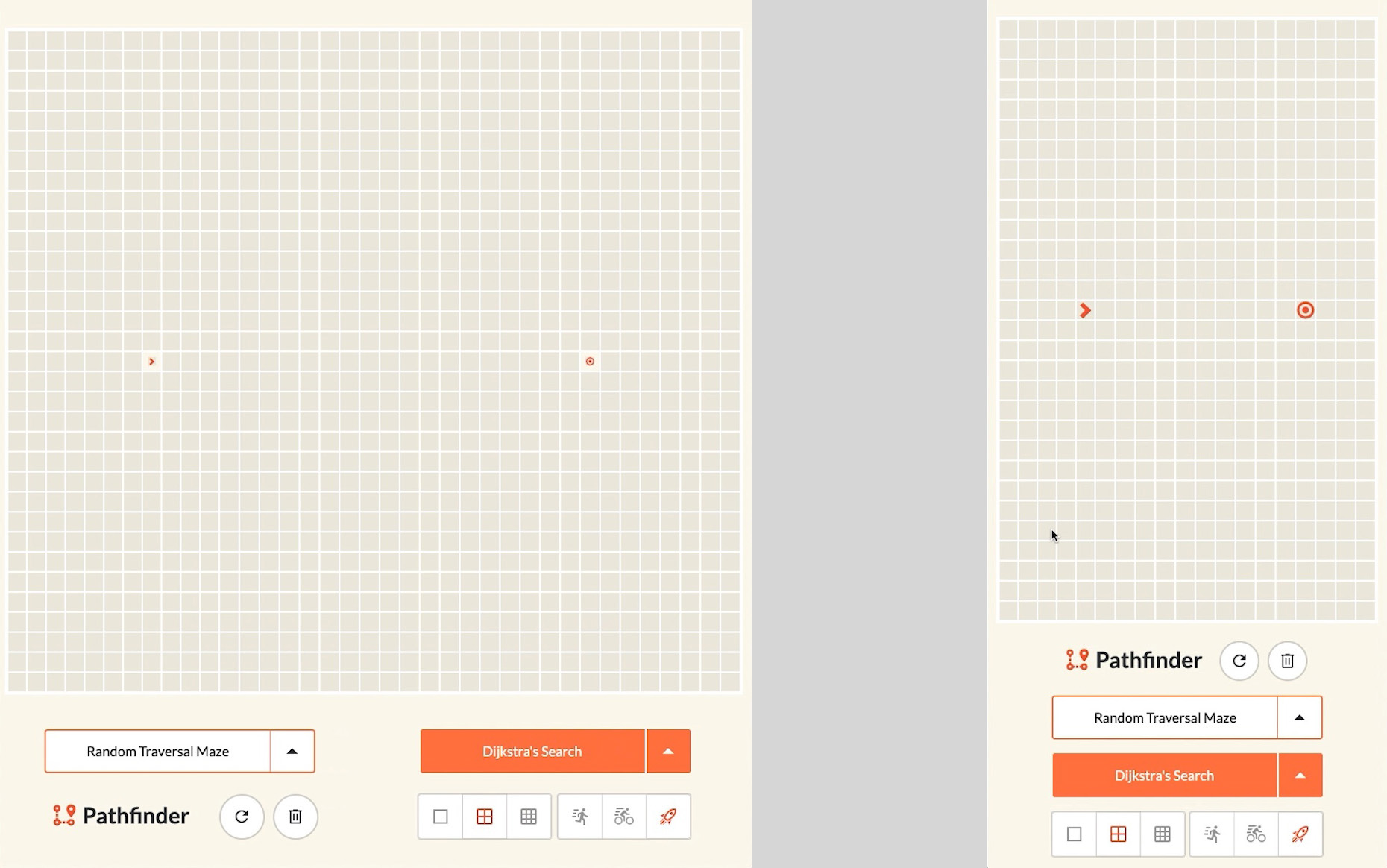
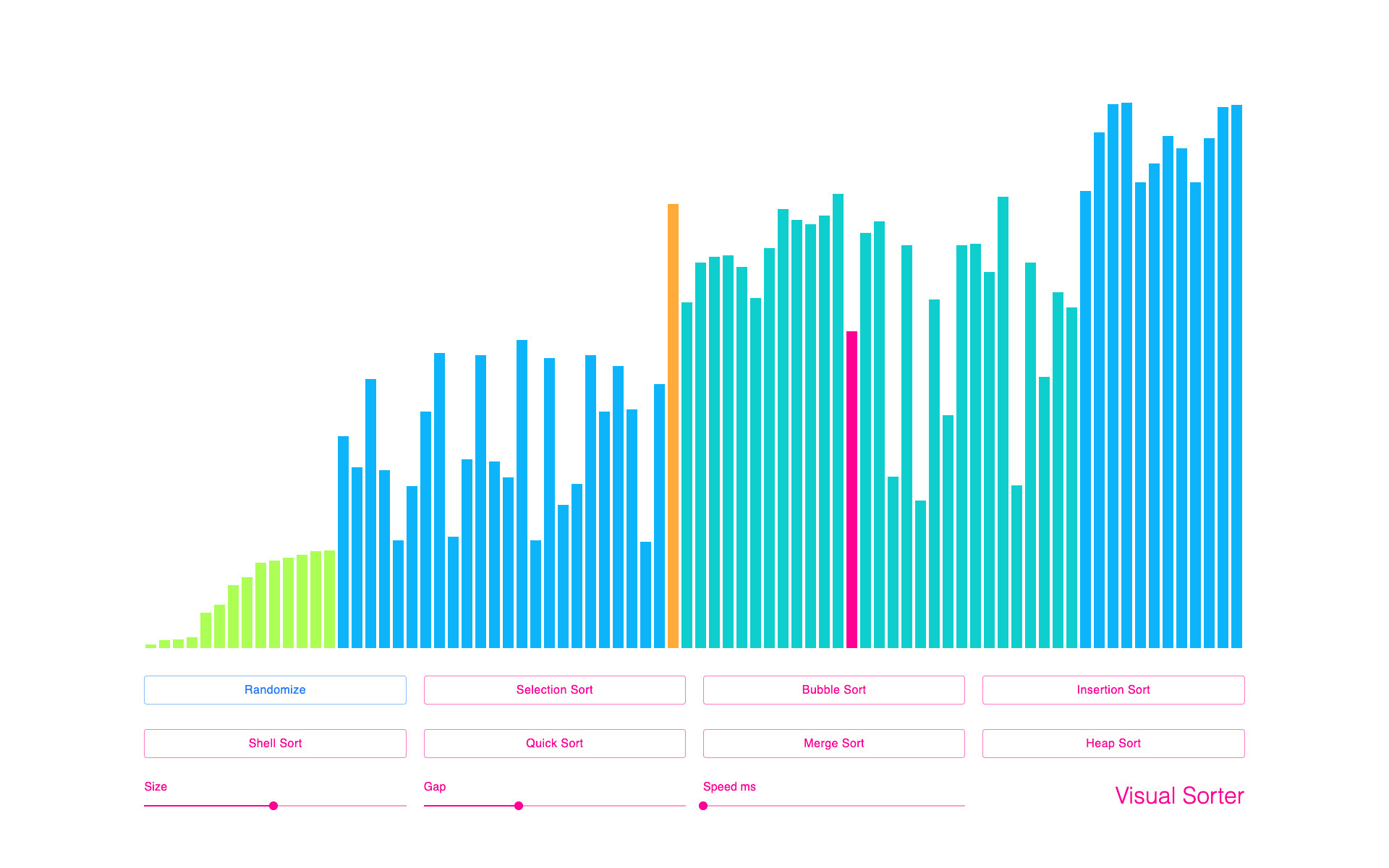
Visual Sorter
Vizualize seven sorting algorithms icluding
Selection, Bubble, Insertion, Shell, Quick, Merge and Heap Sort.
React · JavaScript · Node.js · CSS · HTML · Material Design · UI · UX
Website ↗Sorting Visualizer
Visual Sorter is an application for visualizing sorting algorithms. Built with React and Javascript, application rerenders view updates without the need to reload the page after the change of each state. An await / async functionality is used to slow down the computation and to visualize sorting in slow motion, step-by-step.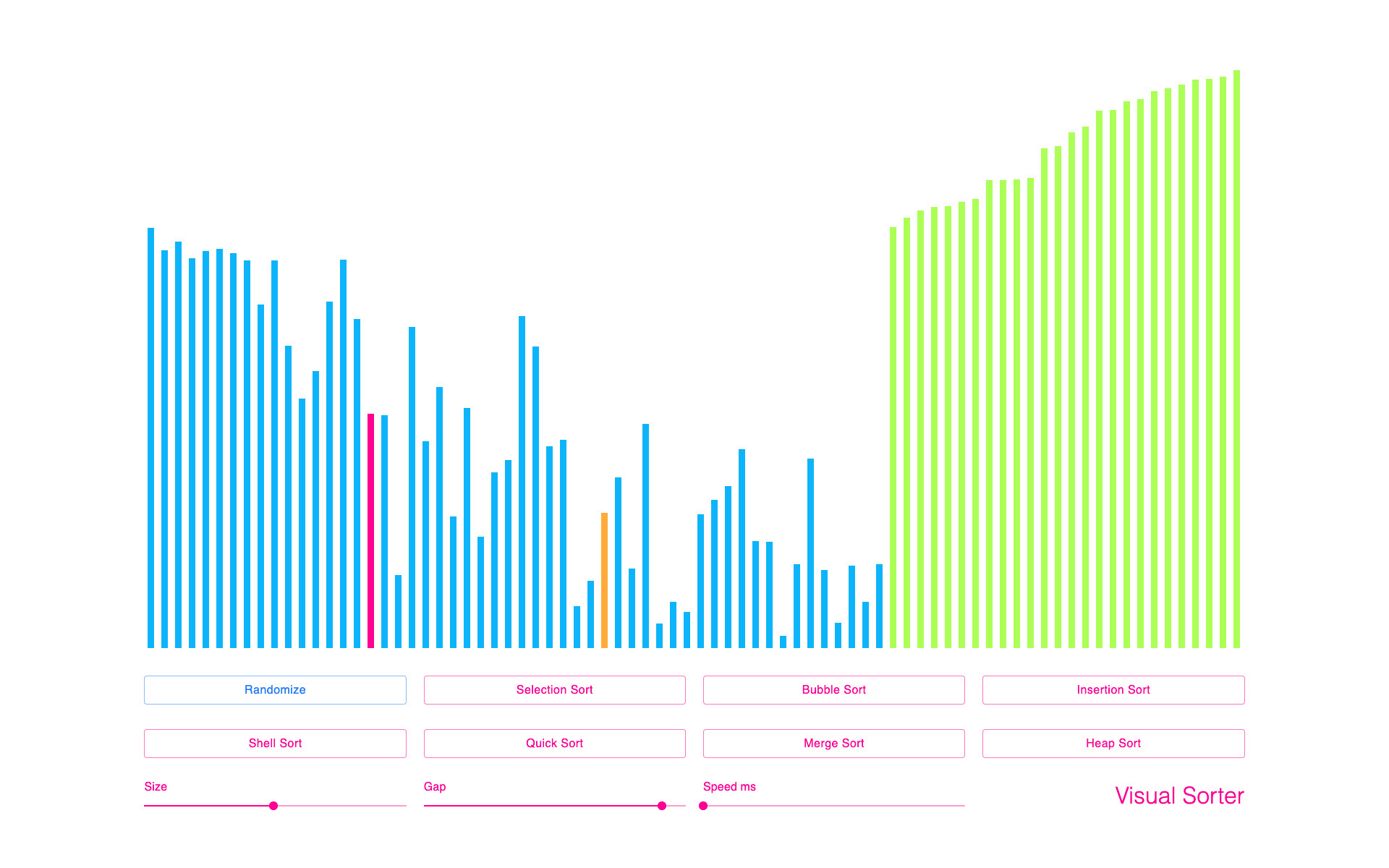
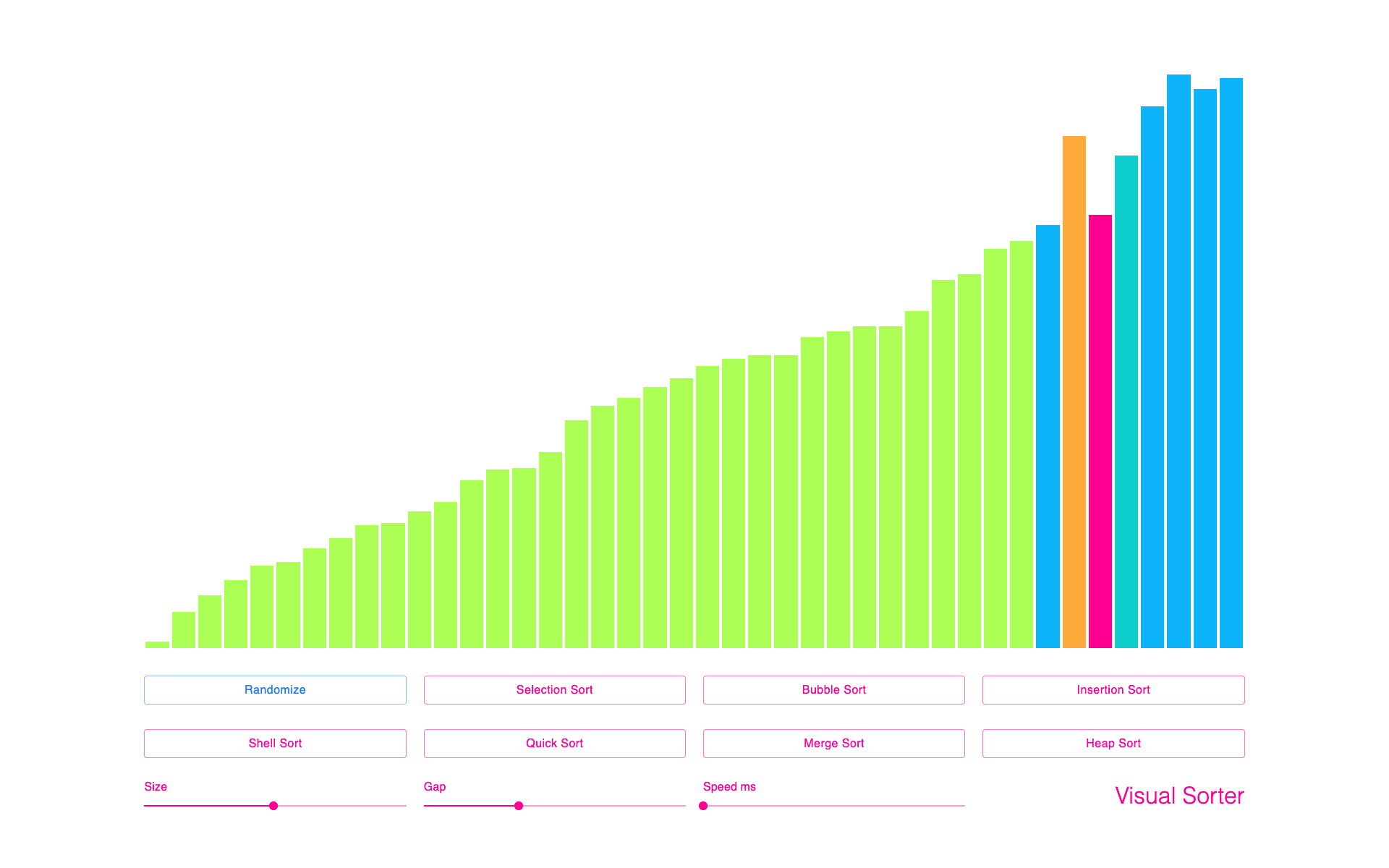
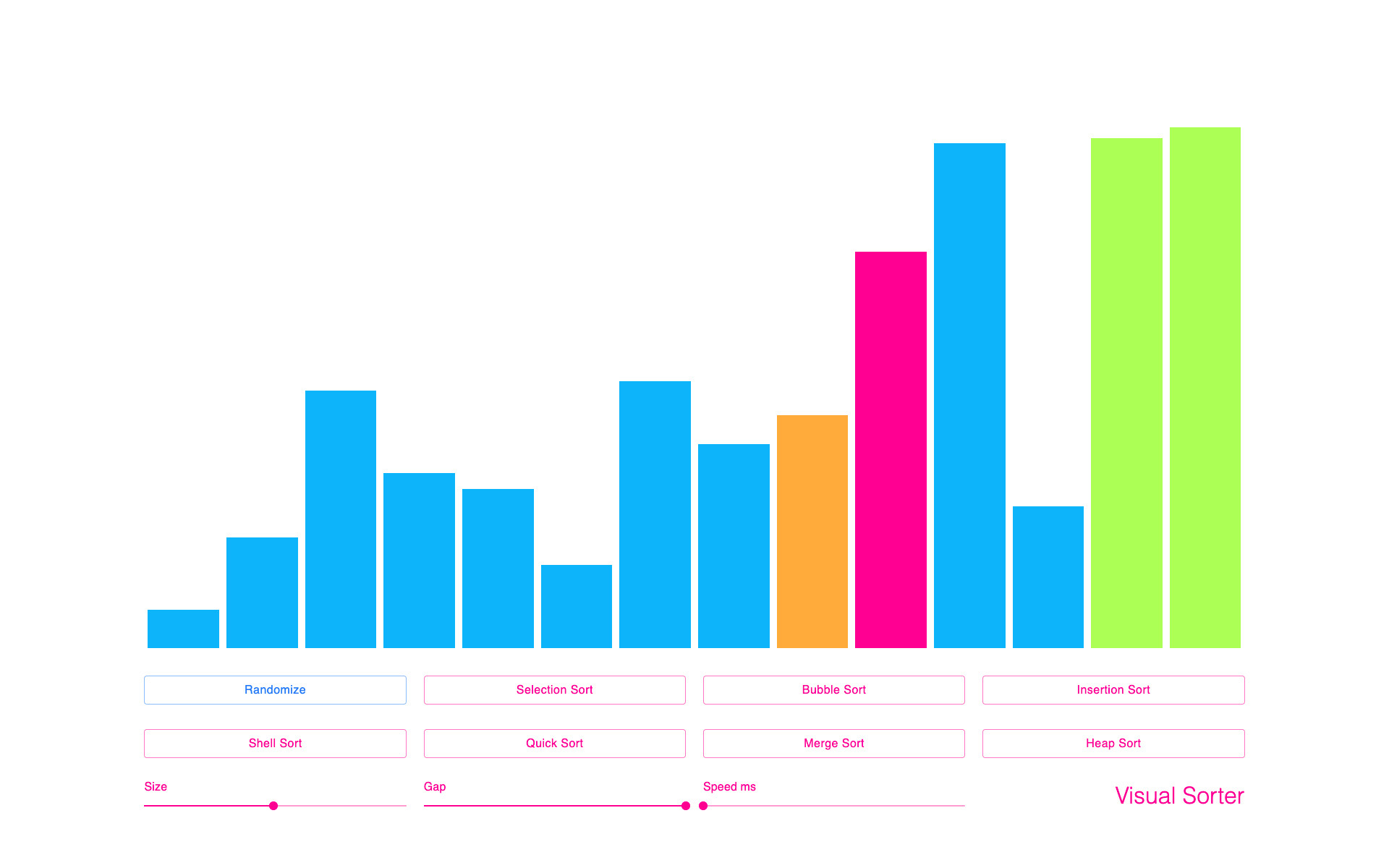
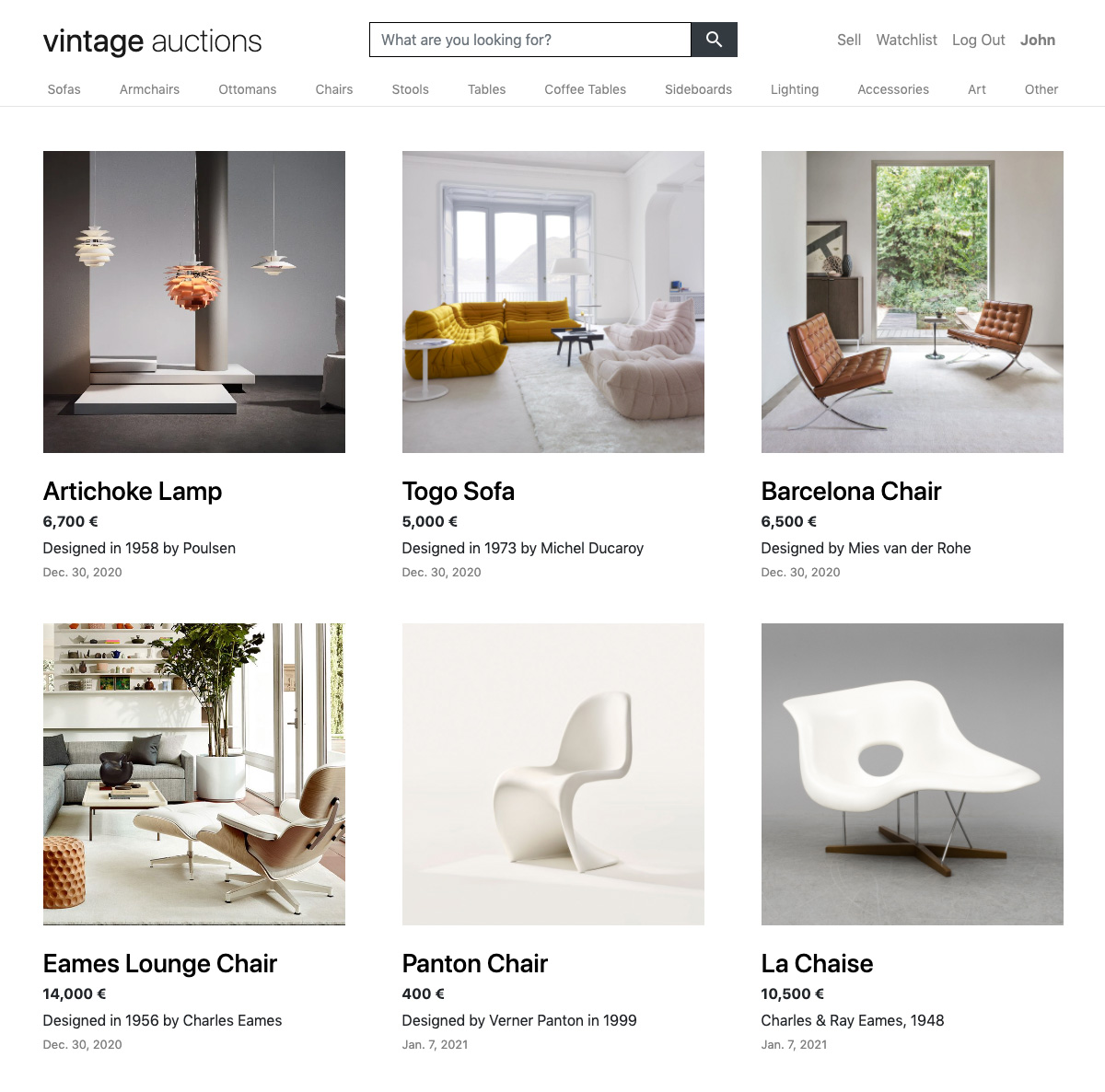
Vintage Auctions
Find and buy trendy classic furniture.
Bid in auction listings or sell your own.
Django · Python · SQL · CSS · HTML · Bootstrap · UI · UX
Website ↗Active listings
Vintage Auctions is an e-commerce auction site that allows users to bid and post auction listings for trendy classic furniture. The front-end of the site is built with a Bootstrap CSS framework directed at responsive, mobile-first web development. The back-end is designed using Django, a high-level Python Web framework that encourages rapid development and clean, pragmatic design by following the model-template-views architectural pattern.Models
The application has multiple Django models such as User, Listing, Bid, Comment, Watchlist and their corresponding Django forms.Create New listing
All the data provided by the user to create a new listing is collected by Django Model Forms and stored at SQLite database.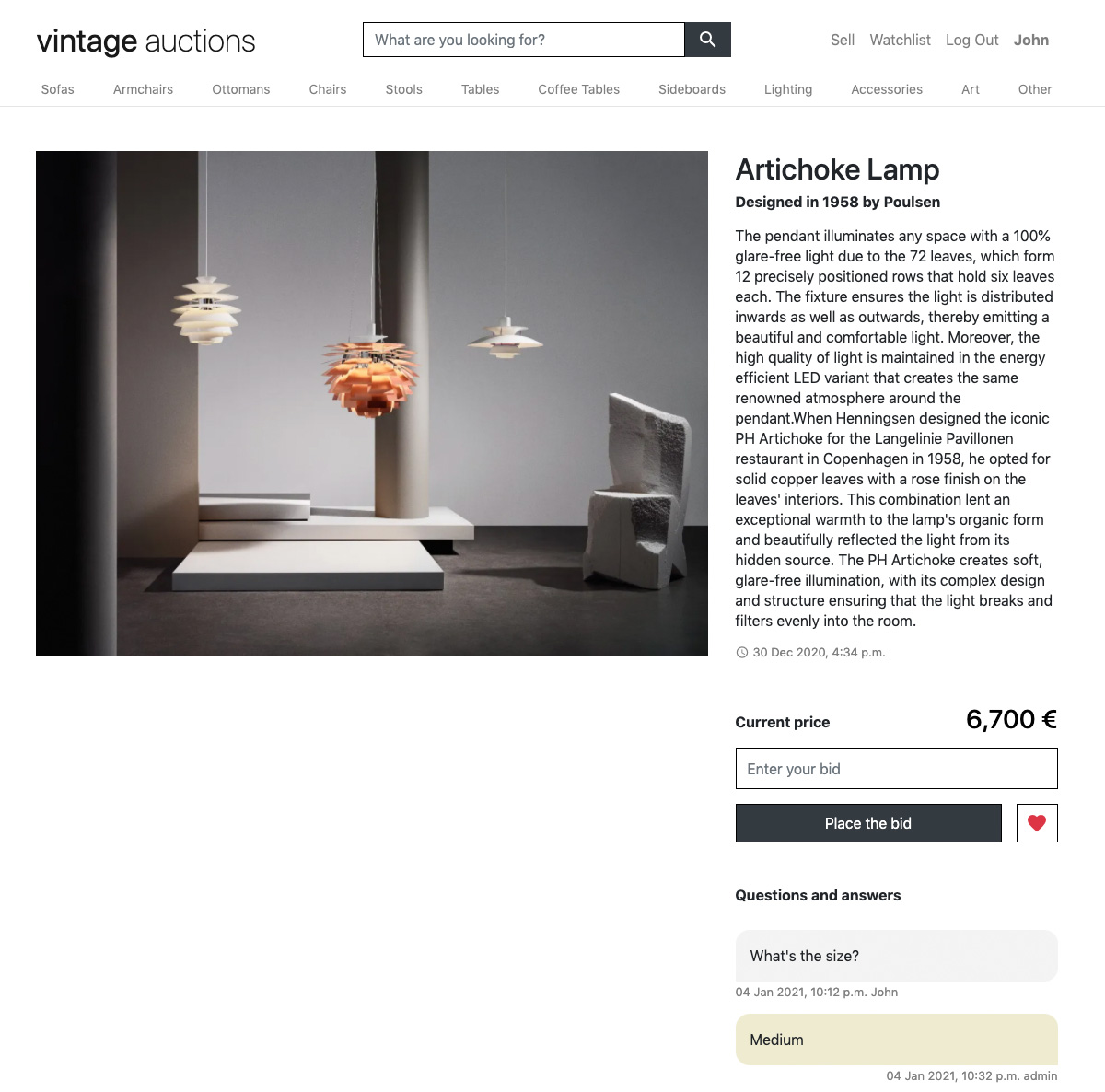
Listing's Page
Listing's page allows users to place bids, comment, and add item to a watchlist. Clicking on a listing takes users to its page, where users are able to view all details about the listing, including the current price. If the user is signed in, the user is able to add the item to their “Watchlist.” If the item is already on the watchlist, the user is able to remove it. If the user is signed in, the user is able to bid on the item. The bid must be at least as large as the starting bid, and must be greater than any other bids that have been placed (if any). If the bid doesn’t meet those criteria, the user is presented with an error. If the user is signed in and is the one who created the listing, the user has the ability to “close” the auction from this page, which makes the highest bidder the winner of the auction and makes the listing no longer active. If a user is signed in on a closed listing page, and the user has won that auction, the page says so. Users who are signed are able to add comments to the listing page. The listing page displays all comments that have been made on the listing.Watchlist
Users who are signed in are able to visit a Watchlist page, which displays all of the listings that a user has added to their watchlist. Clicking on any of those listings takes the user to that listing’s page.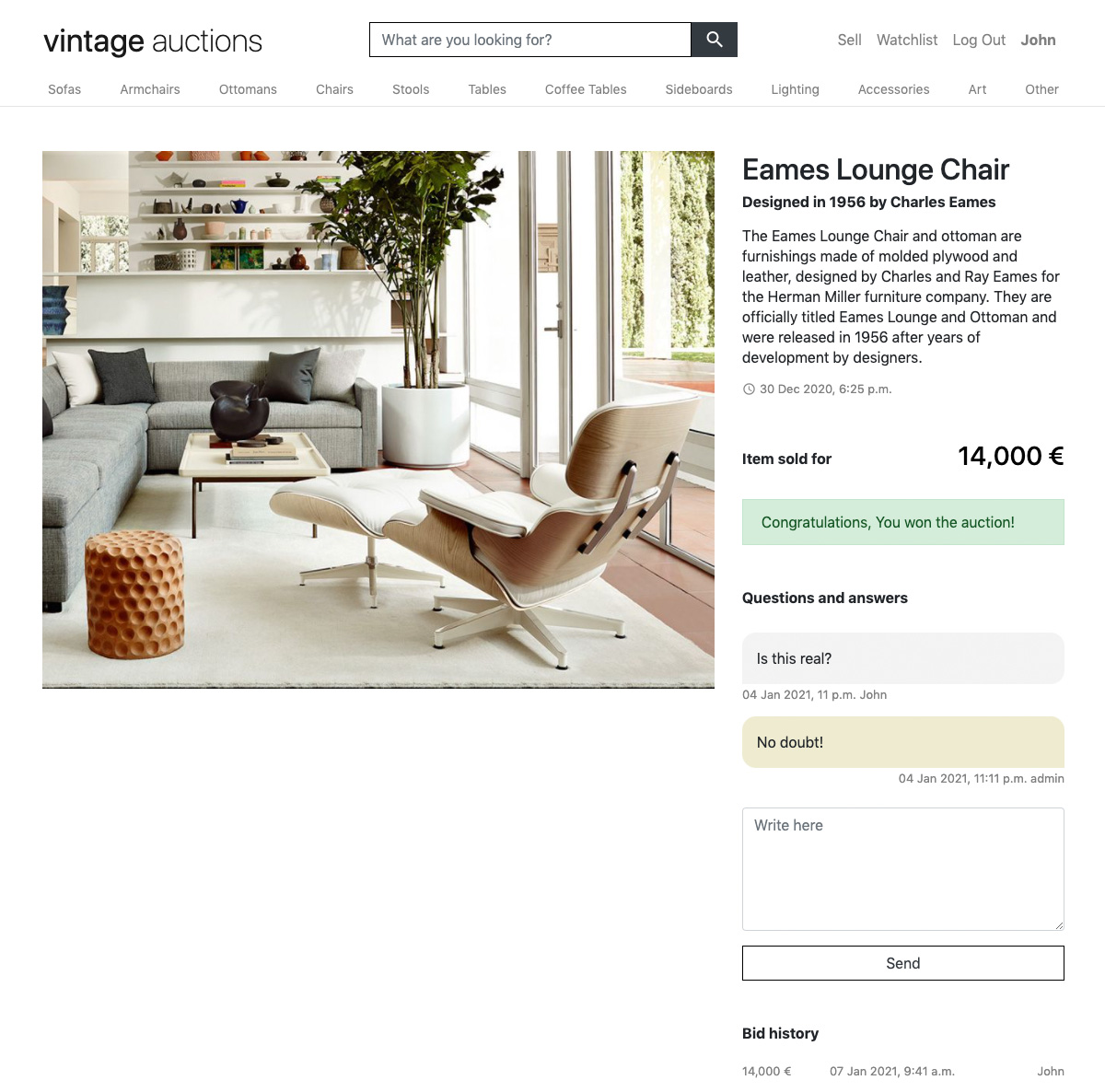
Categories
Clicking on the name of any category takes the user to a page that displays all of the active listings in that category.Mobile first responsive design
The application has a responsive design in all screen sizes, including desktops, tablets and smartphones.Django Admin Interface
Via the Django admin interface, a site administrator is able to view, add, edit, and delete any listings, comments, and bids made on the site.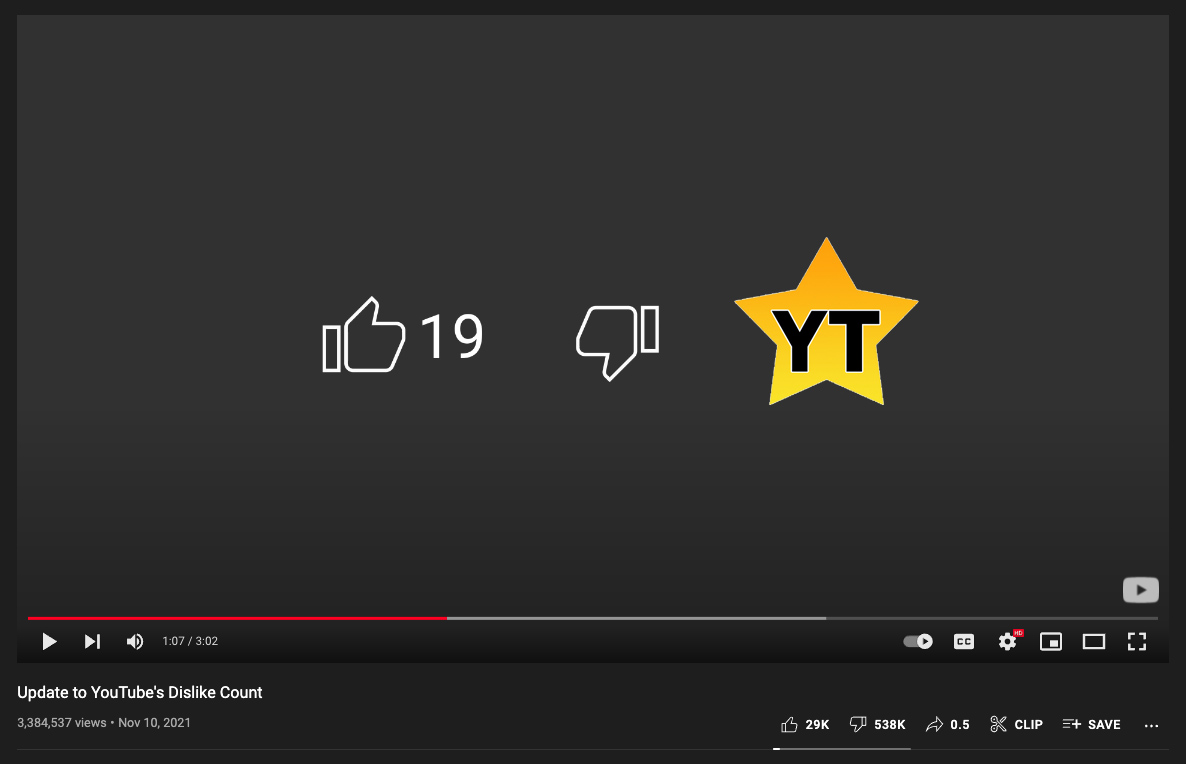
YouTube Rating
Chrome extension that shows YouTube video rating.
Replaces 'Share' button text with a rating like IMDB.
JavaScript · CSS · HTML
GitHub ↗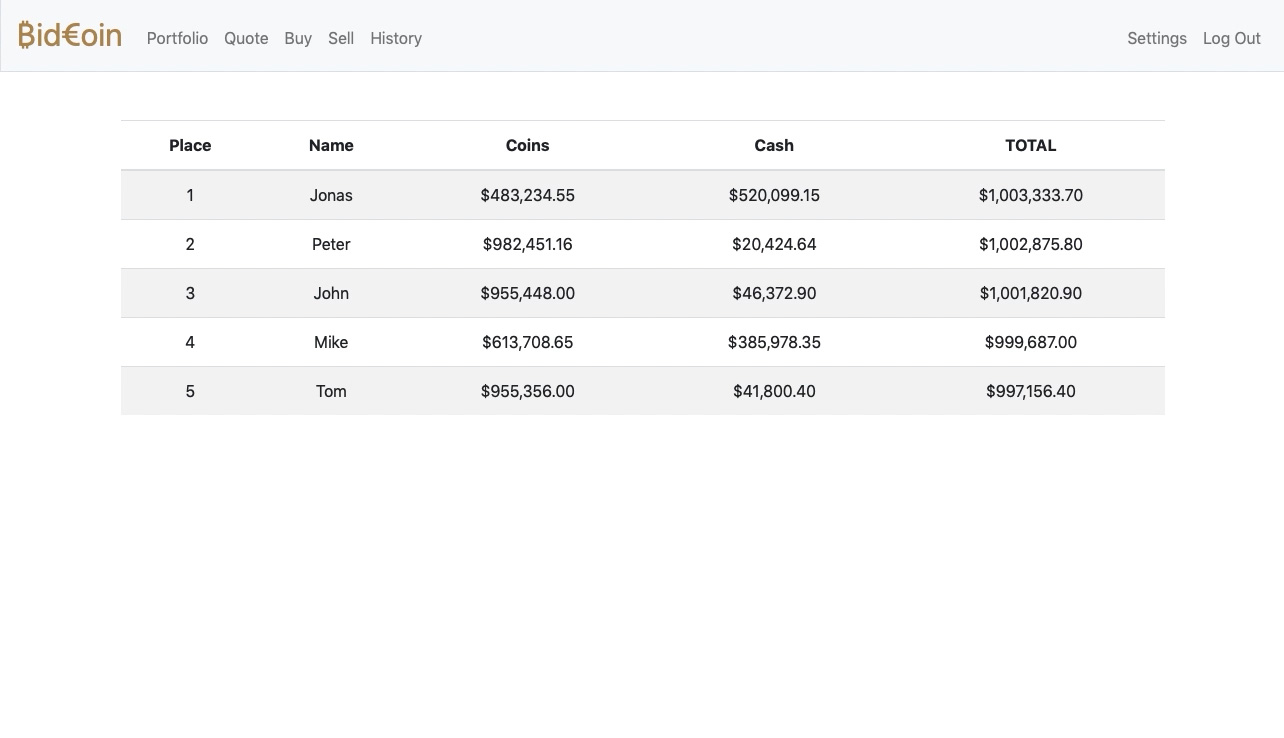
₿id€oin
Try investing in crypto market risk-free.
Compete with your friends or other players.
Flask · Python · SQL · CSS · HTML · Bootstrap
₿id€oin
Built with Python, Flask and SQLite BidCoin is a cryptocurrency simulator game for friends who love to brag how cleverly they would invest into digital coins if they would have a million bucks.
This is the place to prove it and compete with your fellow friends without putting a second mortgage over your house or breaking the bank.
On the other hand one can actually give it a try before investing real money into the cryptocurrency market.Competition
Main page hosts Competition among all the users where visitors can see who is leading the race in profiting from the bullish crypto market.Register
Users are able to register for an account via a form by providing username and password. If username is taken or passwords do not match notification is rendered. Input is submitted via POST to a database where password is stored as a hash.Quote
Quote section allows users to lookup a cryptocurrency’s current price. Once a coin symbol is submitted quotes are downloaded using API in JSON format and the current price is rendered.Buy / Sell
Users can buy or sell shares by providing the cryptocurrency symbol and the number of shares. Transaction execution updates database accordingly.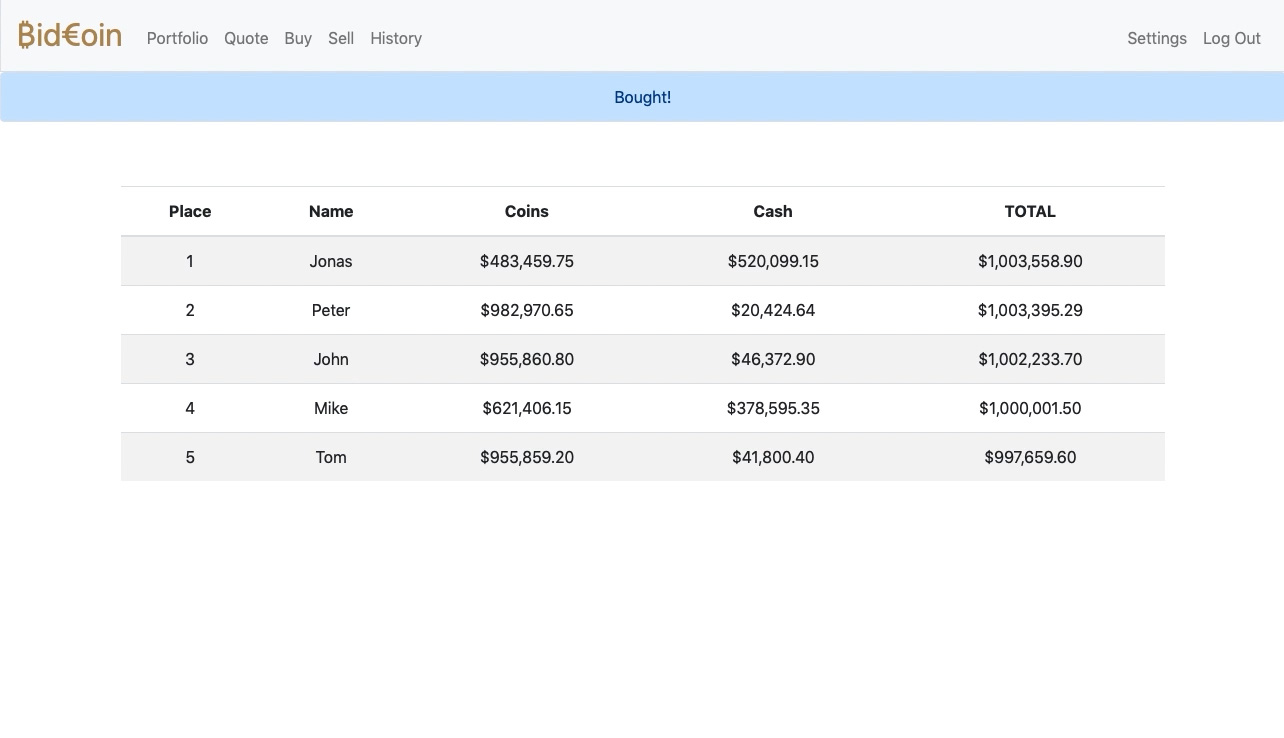
Portfolio
Portfolio section displays an HTML table summarising, which crypto coins the user owns, the current price of each coin, and a total value of each holding. Also displays a current cash balance along with a grand total. Information is retrieved by querying the database.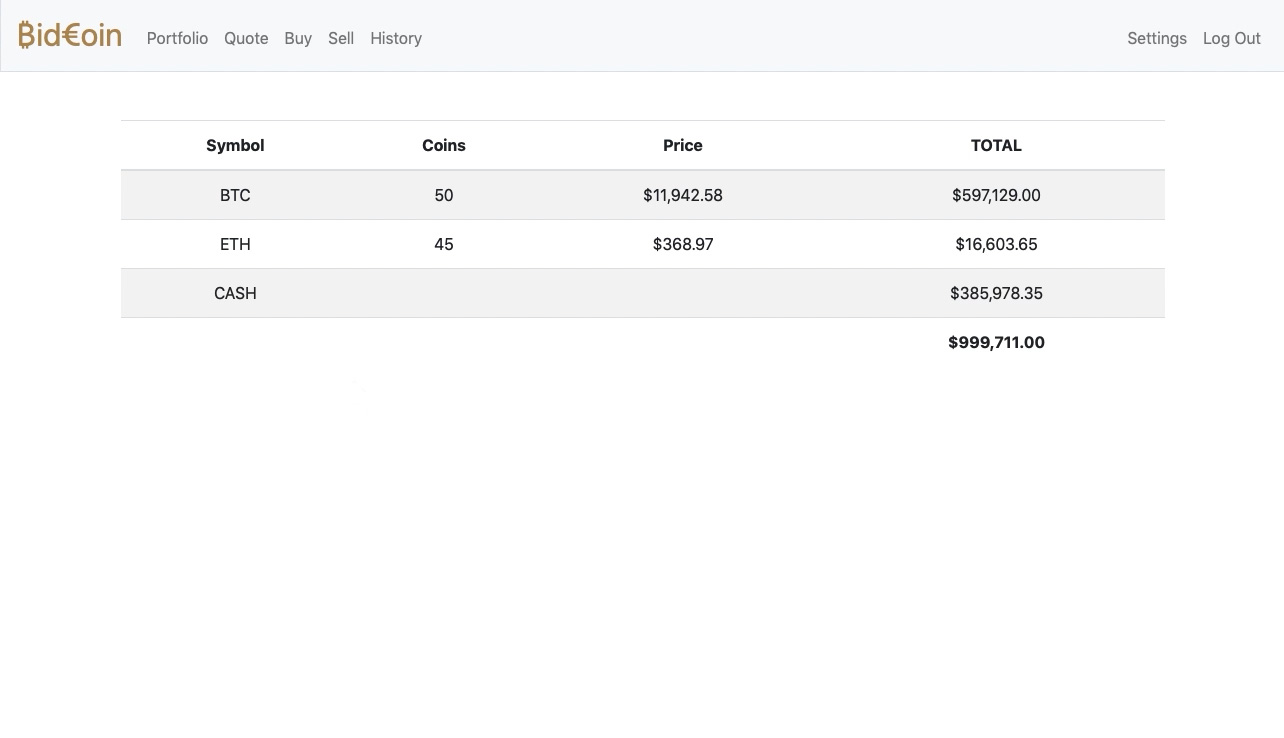
History
History section tracks all the user’s transactions ever, listing row by row each and every buy and every sell. Including coin symbol, price, number of coins sold or bought, and the date and time at which the transaction occurred.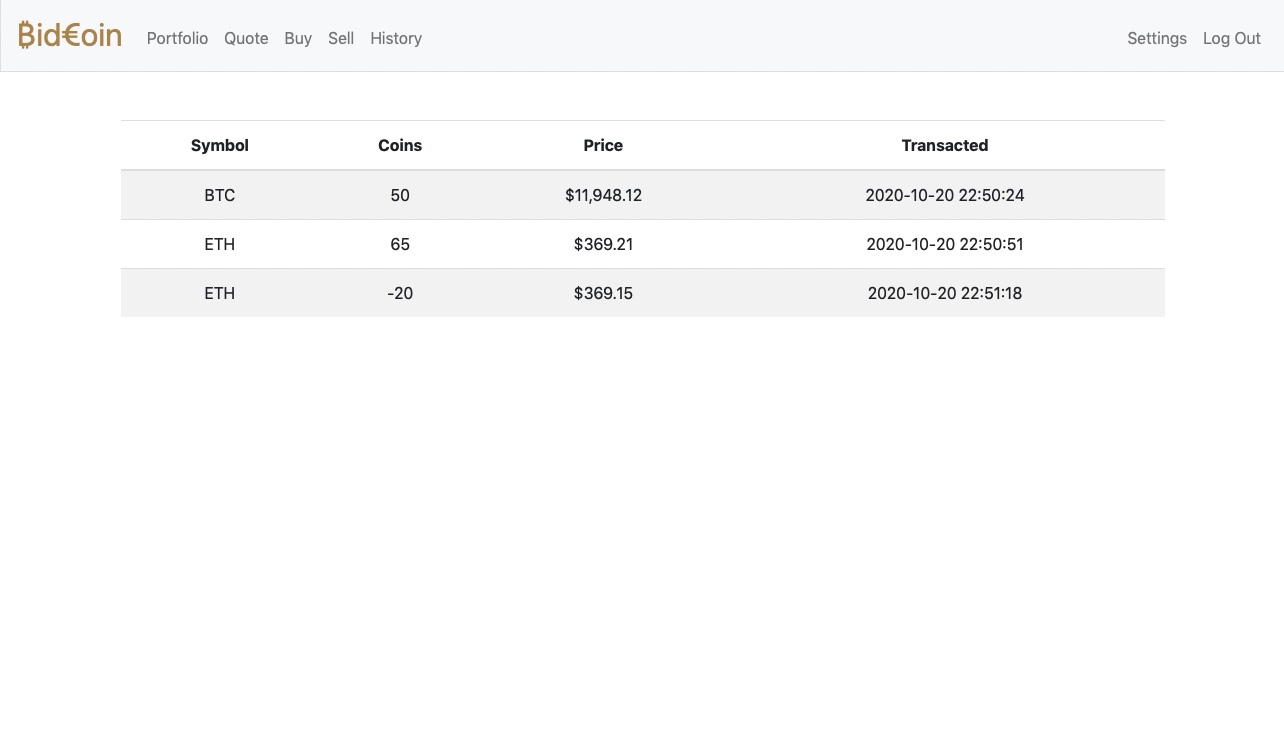
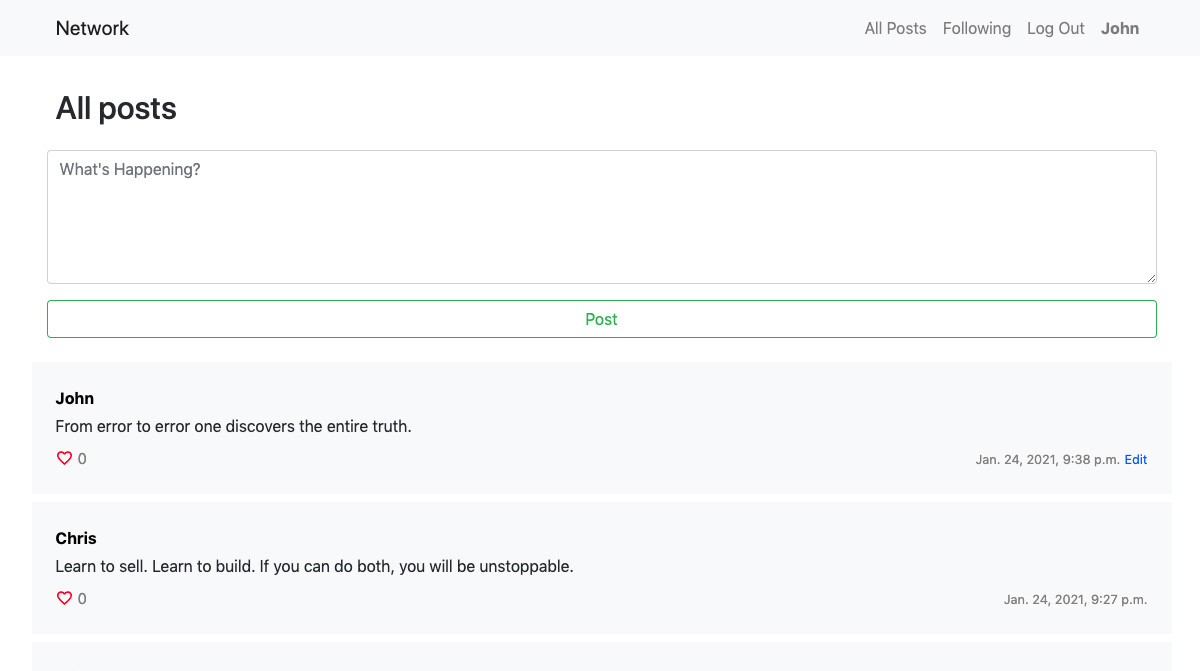
Social Network
Connect with people. Follow influencers.
Build your audience. Share the news and your views.
Javascript · CSS · HTML · Bootstrap
Social network
Built with Python, JavaScript, HTML, and CSS, the implementation of a social network allows users to make or like posts and follow other users.New Post
Users who are signed in are able to write a new text-based post by filling in text into a text area and then clicking a button to submit the post.All Posts
The “All Posts” link in the navigation bar takes the user to a page where they can see all posts from all users, with the most recent posts first. Each post includes the username of the poster, the content itself, the date and time at which it was made, and the number of “likes” it has.Profile Page
Clicking on a username loads that user’s profile page that displays the number of followers the user has, as well as the number of people that the user follows and also displays all of the posts for that user, in reverse chronological order. For any other user who is signed in, this page displays a “Follow” or “Unfollow” button that lets the current user toggle whether or not they are following this user’s posts.Following
The “Following” link in the navigation bar takes the user to a page where they see all posts made by users that the current user follows. This page behaves just as the “All Posts” page does, just with a more limited set of posts and is available only to users who are signed in.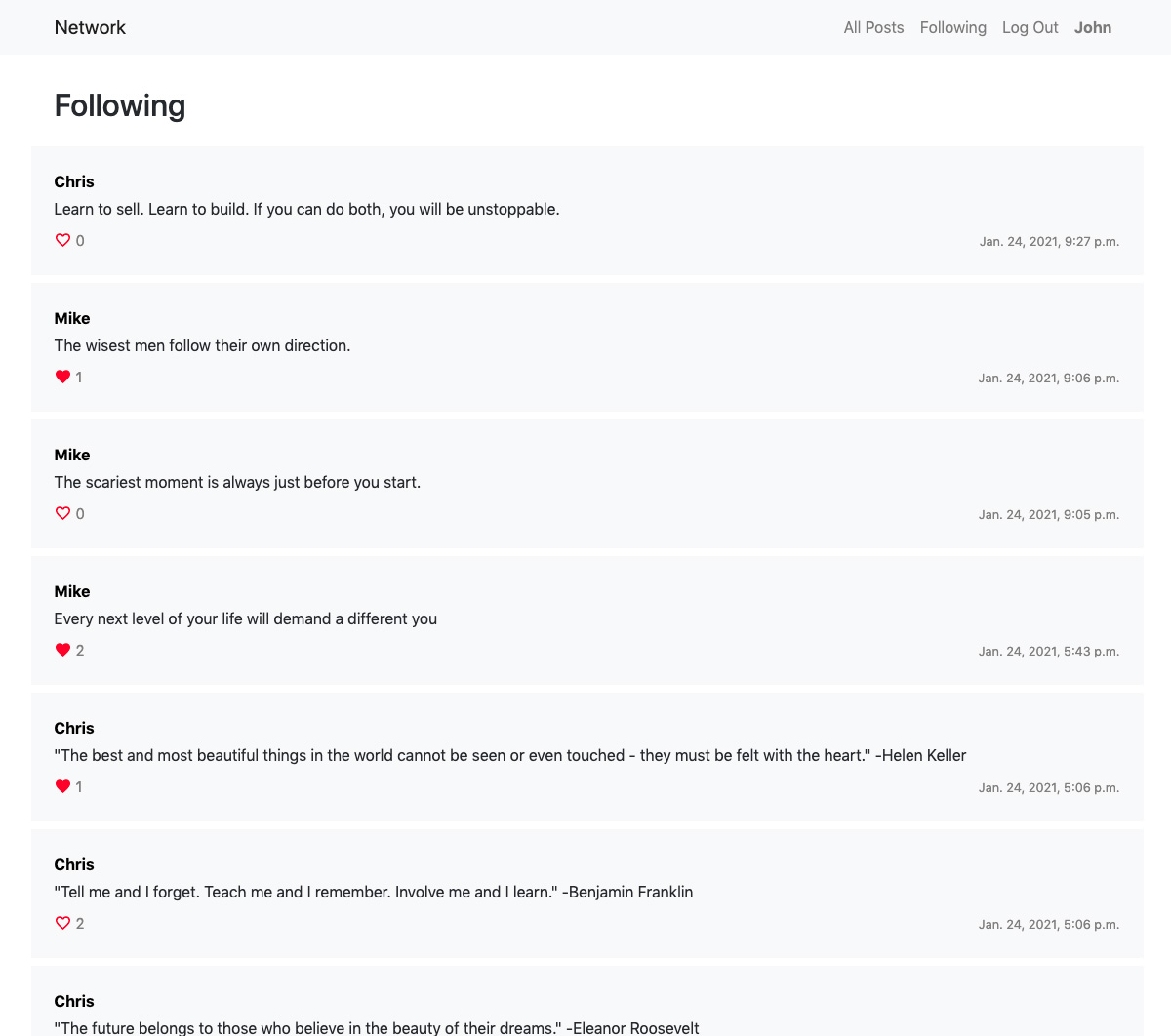
Pagination
On any page that displays posts, only 10 posts are being displayed. Django’s Paginator class is used for implementing pagination on the back-end. Bootstrap’s Pagination features are used for displaying pages on the front-end.Edit Post
When a user clicks “Edit” for one of their own posts, the content of their post is being replaced with a textarea where the user can edit the content of their post. The user is then able to “Save” the edited post. Using JavaScript, this is achived without requiring a reload of the entire page.“Like” and “Unlike”
Users are able to click a button on any post to toggle whether or not they “like” it. Using JavaScript, the server asynchronously updates the like count via a call to fetch and then updates the post’s like count displayed on the page, without requiring a reload of the entire page.Mobile first responsive design
The application has a responsive design in all screen sizes, including desktops, tablets and smartphones.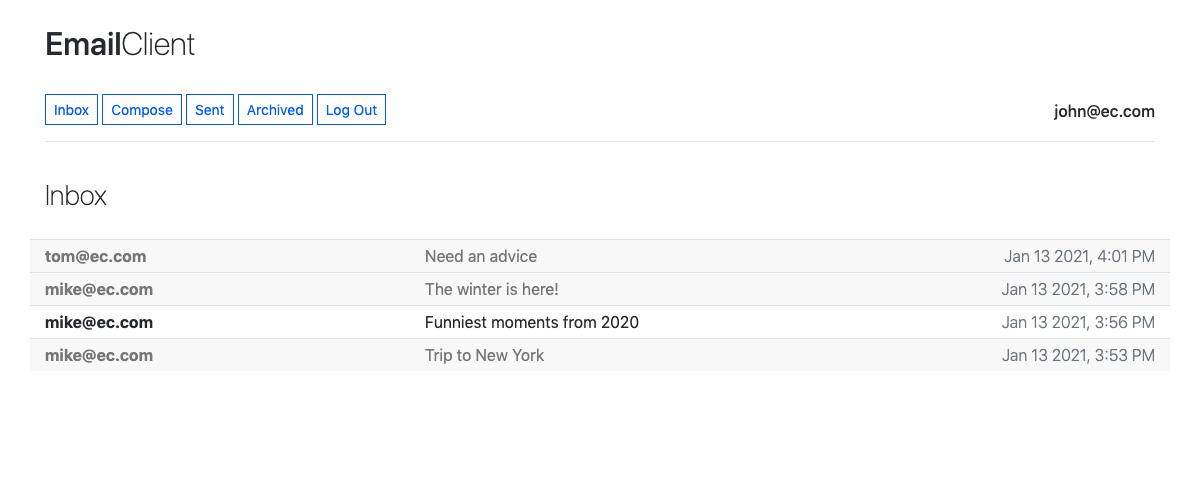
Email Client
Keep your mailbox light and simple.
Yet have all the functionality that you need.
Javascript · CSS · HTML · Bootstrap
EmailClient
EmailClient is a front-end for an email client that makes API calls to send and receive emails. The app is built mainly with Javascript on top of Python's framework Django.Send Mail
When a user submits the email composition form, a POST request passes values of recipients, subject and body to /emails route. Once email has been sent, user's SENT mailbox is loaded.Mailbox
When a user visits their INBOX, SENT mailbox or ARCHIVE, the appropriate mailbox is loaded from JSON response. When a mailbox is visited, the application first queries the API for the latest emails in that mailbox. Each email is then rendered in its own 'div' box that displays who the email is from, what the subject line is, and the timestamp of the email. If the email is unread, it appears with a white background. Otherwise with a gray background and muted text.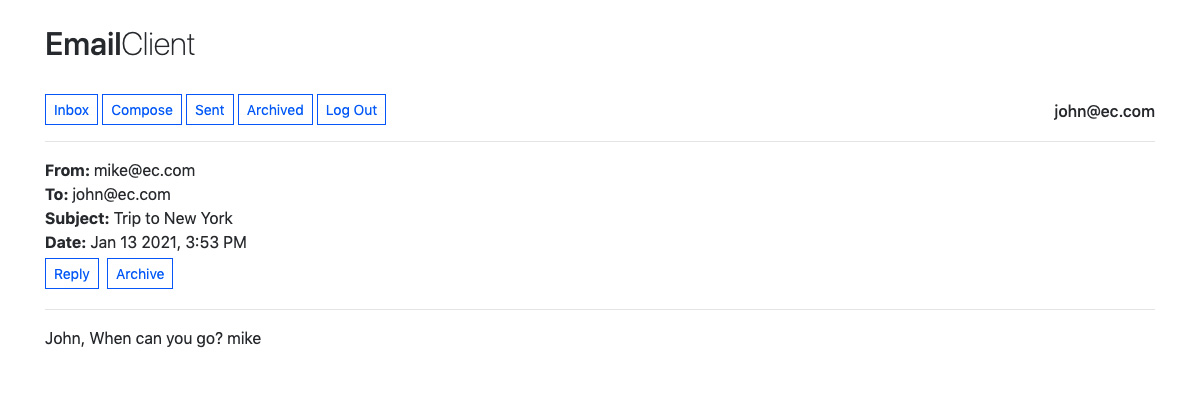
View Email
When a user clicks on an email, the user is taken to a view where they see the content of that email. It shows the email’s sender, recipients, subject, timestamp, and body. Once the email has been clicked on, the email is marked as read. Additional 'div' element is added for displaying the email. Code hides and shows the right views when navigation options are clicked.Archive and Unarchive
When viewing an email, the user is presented with a button that lets them archive or unarchive the email. Once an email has been archived or unarchived, the user’s inbox is loaded.Reply
When viewing an email, the user is presented with a “Reply” button that lets them reply to the email. When the user clicks the “Reply” button, they are taken to the email composition form. The composition form is pre-filled with the recipient field set to whoever sent the original email, the subject line and the body of the email with details of the original email.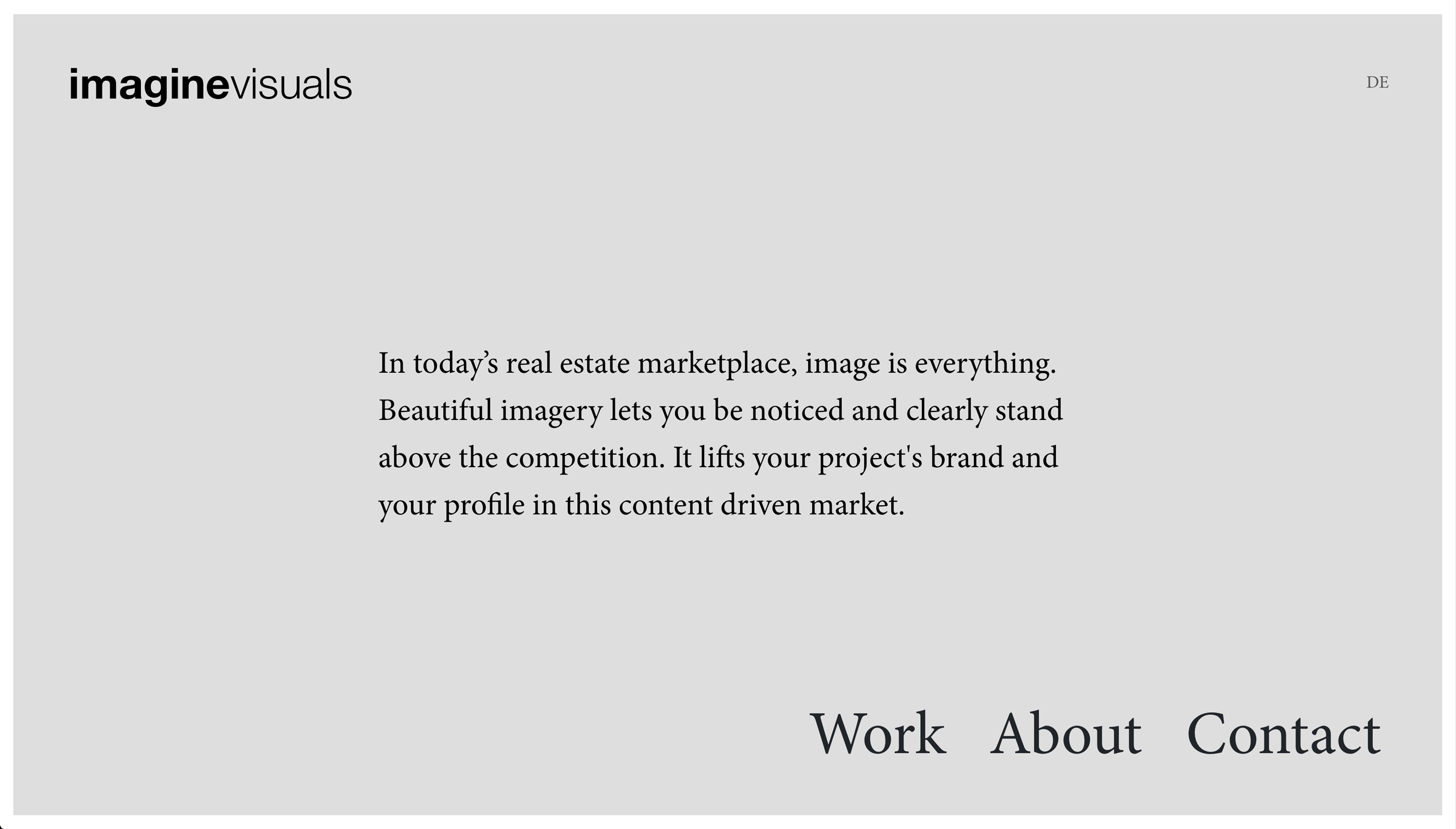
Portfolio Website
Website for a visualisation studio.
Visualise architecture.
React · Gatsby · Javascript · HTML · CSS · UI · UX
Website ↗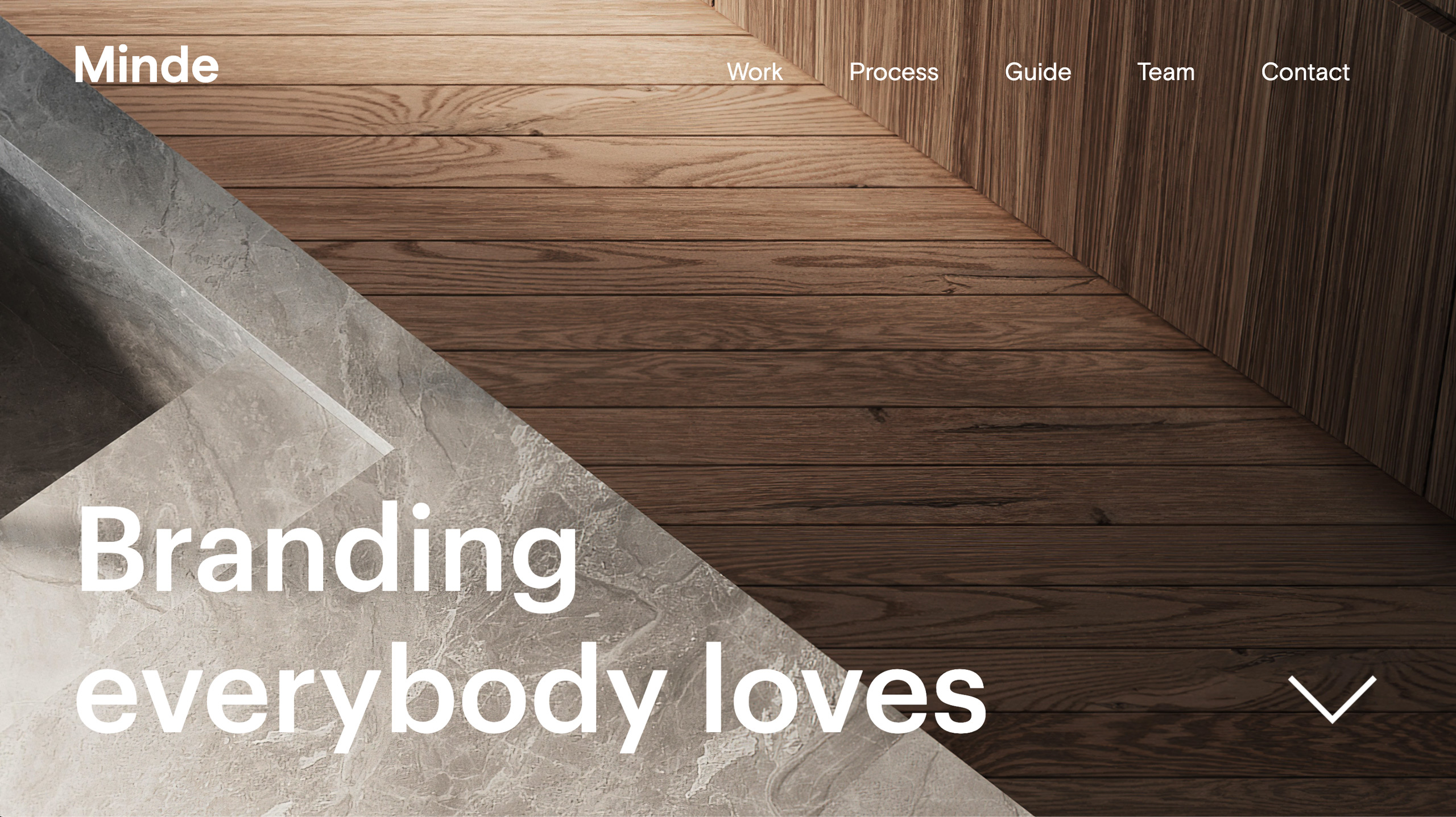
Startup Website
Website for a branding agency.
Create a brand for architecture.
UI · UX · Webflow · HTML · CSS
Website ↗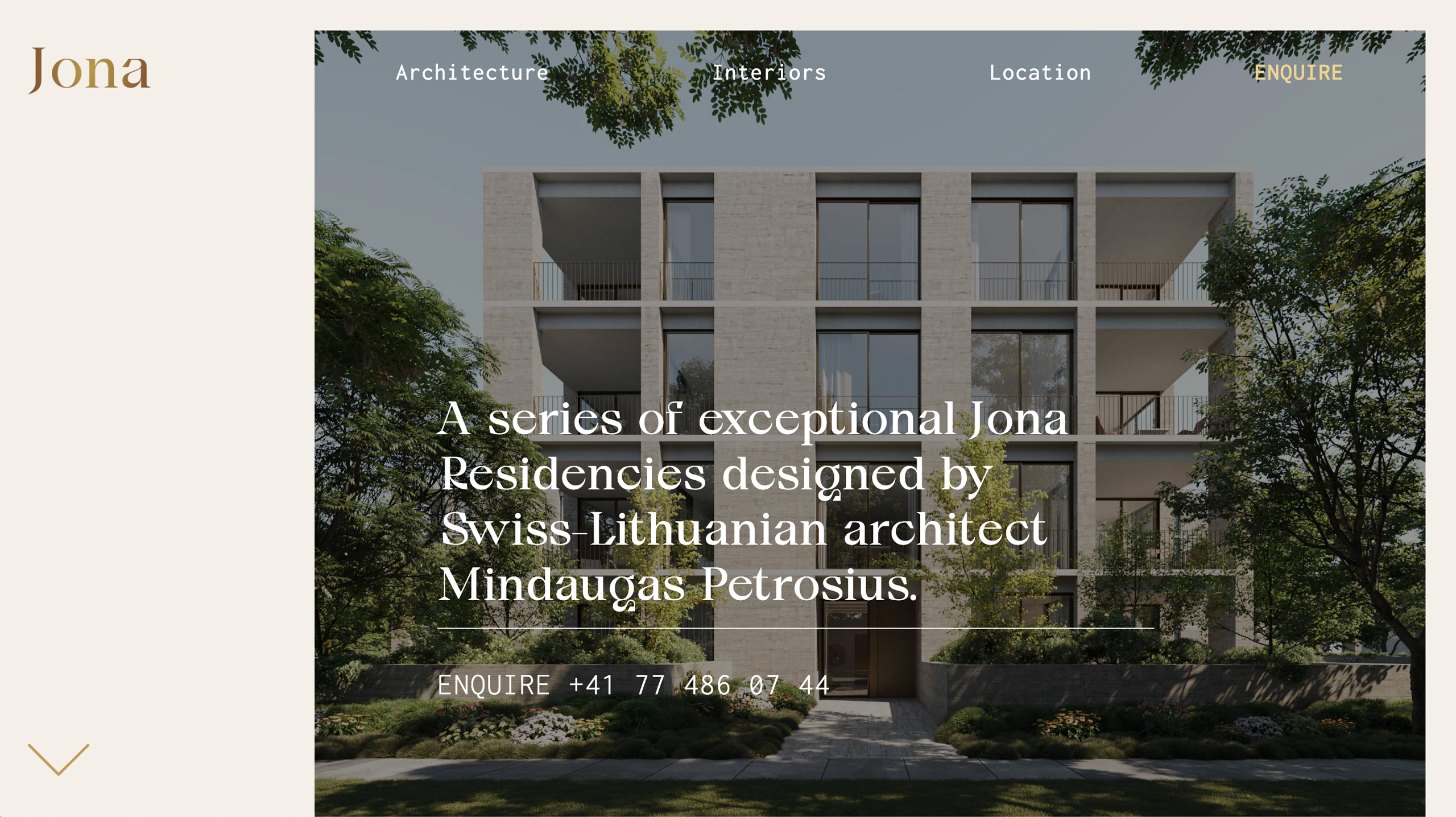